Place Your Query
C Programming
- Control flow-based C Programs
- Enjoy Conditional Programming in C using If...else and switch statement.
- Good, Now write a C program to display "Hello World" on the screen.
- Write a C program to display Your Name, Address and City in different lines.
- C program to find sum of n numbers
- Write a C program For Addition of two numbers using Function.
- Write a Program to Find Simple Interest and Compound Interest.
- Write a Program to Convert Celsius To Fahrenheit and Vice Versa.
- Write a Program to Find Area and Perimeter of Circle, Triangle, Rectangle and Square.
- Write a Program to Swap Two Numbers Using Temporary Variables and Without Using Temporary Variables
- Write a C Program to Design a Simple Menu Driven Calculator
- Simple Expression Based C Programs
- 7. Components of C language
- 1. Introduction to C Programming Language
- 10. Operator Precedence and Associativity
- 11. Comments in C Programming
- 14. Fundamental Control Structure Statements in C [Part-1]
- 15. Fundamental Control Structure Statements in C [Part-2]
- 16. Looping Statements [Fundamental Control Structure Statements in C. #Part-3]
- 17. Keyword break, continue, return and exit [Fundamental Control Structure Statements in C. #Part-4]
- 2. Computer Languages
- 3. Interpreters vs Compilers vs Assemblers in programming languages
- 4. C Program Structure
- 5. Compile and Execute C Program
- 6. Errors in C Program
- 8. C Datatypes
- 9. Operators in C
- Control flow-based C Programs
- Demystifying Bit Masking: Unlocking the Power of Bitwise Operators in C-Language with best Examples.
- 18. Fundamentals of C Functions
- Show Remaining Articles (3)Collapse Articles
Java
AI ML
FAQs
- A Program to find Tokens in C code?
- What are the Common Coding Mistakes.
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
Python Interview Questions
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
6. Errors in C Program
What is an error in a c program?
An error is an illegal operation performed by the user which results in abnormal working of the program. Programming errors often remain undetected until the program is compiled or executed. Some of the errors inhibit the program from getting compiled or executed. Thus, errors in a c program should be removed before compiling and executing.
It is important for programmers to thoroughly test their code and identify any potential errors before compiling and executing the program. This can help prevent unexpected issues and ensure the smooth functioning of the program. Additionally, using debugging tools and techniques can help in identifying and fixing errors in the code.
Some errors in C are hidden or prevent the program from being compiled or executed. So while executing our application successfully, we must remove the errors from the program.
Real-Time Scenario:We have an application for displaying the sum of the numbers while declaring variables; we have missed the semicolon or wrong syntax of the main method, resulting in an error while executing the application.
Types of Errors in C
The most common errors can be broadly classified into five different types, as follows:
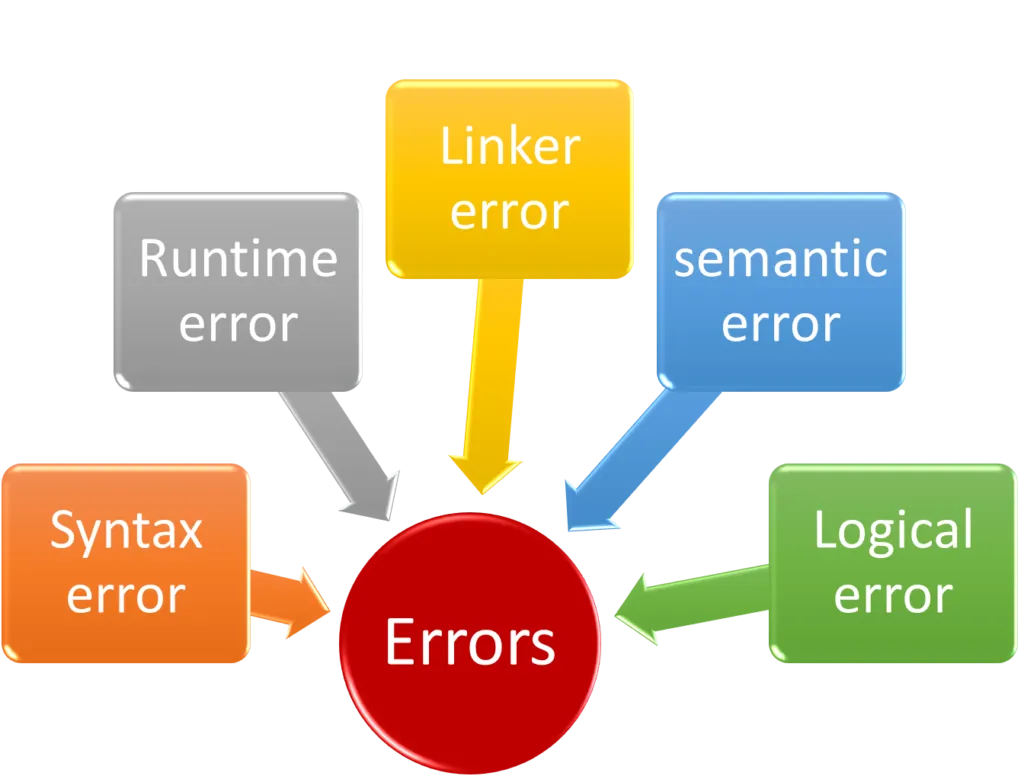
1. Syntax Errors
Errors occur when you violate the rules of writing C syntax is said to be “Syntax errors.” This compiler error indicates that it has to be fixed before the code will be compiled. The compiler identifies these errors as “compile-time errors.”
Example 1
void main()
{
int a //<-- you slipped to close the statement or sentence by placing semi colon(;) at the end.
}
Example 2
void main()
{
int x;
// In this case user has missed the clossing parenthesis(})
2. Run-Time Errors
Errors that occur after a successful compilation of the program are referred to as “run-time errors.” Dividing a number by zero, going out of bounds in an array or a string, and similar issues are the most common run-time errors. These errors are generally difficult to detect during the compile time.
Example 1
void main()
{
int a=10;
int c=a/0;//<-- In this case you will get an error of number divisible by zero error occurs
}
Example 2
#nclude<stdio.h>
void main()
{
int a[3]={1,2,3};
int res=a[4]; // <-- Here array index out of bounds error occurs. index will range from 0 to 3 only.
}
3. Linker Errors
These errors are generated after compilation; we link the different object files with the main’s object using the Ctrl+F9 shortcut key. These errors occur when the executable program cannot be generated. This may be because of wrong function declaration, importing incorrect header files, etc. Most frequent linker error is writing Main() instead of a main() method.
Example
void Main() // <-- In this case user typed Main() method, instead of main() function { } so linker fails to link the program for its execution.
4. Logical Errors
If our expectation is one thing and the resulting output is another, then that kind of error we call “Logical error.” Suppose we want the sum of the two numbers, but the given output is the multiplication of 2 digits; this is a Logical error. Detecting such errors is possible by performing line-by-line debugging.
Example
void main()
{
printf("%d",add(10,20));
}
int add(int x, int y)
{
return x*y; //<-- Here expectation of addtion will not be done by multiplying the numbers.
}
5. Semantic Errors
The C compiler generates this error only when the written code is in an incomprehensible format.
Example
void main() { int x, y, z; x + y = z; //semantic error }
Examples of Types of Errors in C
Following are the few examples that shows the occurrence of common errors in a program:
a. Syntax Error with Semicolon Example
Example
#include //Used to include basic c library files
void main() //Used to execute the C application
{ //declaring and defining the variables
int x = 10;
int y = 15; //displaying the output
printf("%d", (x, y)) //<--You missed here to put semi-colon, this will show an error at line no. 6
}
Output:

b. Syntax Error with Mustache Brace Example
Example
#include //Used to include basic c library files
void main() //Used to execute the C application
{
//declaring and defining the variables
int x = 100;
int y = 150;
//displaying the output
printf("%d %d",x,y);
//<-- clossing brace is missing
Output:

c. Run-Time Errors with Array Index out of Bounds Example
Example
#include //Used to include basic c library files
void main() //Used to execute the C application
{
//declaring and defining the array variables
int a[5] = {100,101,102,103,104};
int b[5] = {105,106,107,108,109};
//displaying the output
printf("%d\n",a[100]); //array index out of bounds run-time error
//in c this is not shown any error message it will just show out of bound values as 0
printf("%d\n",b[700]);//garbage value in return
}// end main
Output:

d. Run Time Error with Zero Divisible by Number Example
Example
#include //Used to include basic c library files
void main() //Used to execute the C application
{
//declaring and defining the variables
int x = 200;
int y = 400;
float a=x/10;
float b=y/0;
//displaying the output
printf("%f\n",a); // No error occurs at this statement.
printf("%f\n",b); //This time you will get divide by zero run time error
} // end main
Output:

e. Linker Error with Wrong Main() Method Syntax Example
Example
#include //Used to include basic c library files
void Main() //Linker error as wrong syntax of main method used
{
//declaring and defining the array variables
char a[] = "Milind";
char c[] = "Bhatt"; //displaying the output
printf("%s\n",a);
printf("%s\n",c);
}// end main
Output: Can’t execute

f. Logical Error Example
Example
#include //Used to include basic c library files
int sum(int a, int b);// Including method
void main()//main() method for executing the application
{
//declaring and defining the variables
int a=100;
int b=200;
//displaying the output
printf("Sum of %d and %d is=%d\n",a,b,sum(a,b));//sum(a,b) is calling method
} //end main
//called method
int sum(int a, int b)
{
return a*b;//instead of sum here programmer make a mistake by return multiplication logic
}// end function
Output:

g. Sematic Error Example
Example
#include //Used to include basic c library files
void main() //main() method for executing the application
{
//declaring and defining the variables
int a=10;
int b=20;
int a+b=c;//<-- Here you find a Sematic error by unkwoning c language code
//displaying the output
printf("%d %d",a,b);
}// end main
Output:

Conclusion
Mistakes in the C language arise from writing statements that the compiler cannot understand; as a result, the compiler generates errors. These errors can be due to programmer mistakes or, at times, insufficient memory in the machine to load the code. There are primarily 5 types of errors: Syntax errors, Run-time errors, Linker errors, Logical errors, and Logical errors.Syntax errors occur when the rules of the language are not followed. These are usually caught by the compiler during the compilation process. Run-time errors happen while the program is running and can cause it to terminate abnormally. Linker errors occur when the linker cannot find the necessary library or object files. Logical errors, also known as semantic errors, result in the program performing differently from what the programmer intended. Lastly, logical errors refer to inconsistencies or contradictions within the program’s logic.