Place Your Query
C Programming
- Control flow-based C Programs
- Enjoy Conditional Programming in C using If...else and switch statement.
- Good, Now write a C program to display "Hello World" on the screen.
- Write a C program to display Your Name, Address and City in different lines.
- C program to find sum of n numbers
- Write a C program For Addition of two numbers using Function.
- Write a Program to Find Simple Interest and Compound Interest.
- Write a Program to Convert Celsius To Fahrenheit and Vice Versa.
- Write a Program to Find Area and Perimeter of Circle, Triangle, Rectangle and Square.
- Write a Program to Swap Two Numbers Using Temporary Variables and Without Using Temporary Variables
- Write a C Program to Design a Simple Menu Driven Calculator
- Simple Expression Based C Programs
- 7. Components of C language
- 1. Introduction to C Programming Language
- 10. Operator Precedence and Associativity
- 11. Comments in C Programming
- 14. Fundamental Control Structure Statements in C [Part-1]
- 15. Fundamental Control Structure Statements in C [Part-2]
- 16. Looping Statements [Fundamental Control Structure Statements in C. #Part-3]
- 17. Keyword break, continue, return and exit [Fundamental Control Structure Statements in C. #Part-4]
- 2. Computer Languages
- 3. Interpreters vs Compilers vs Assemblers in programming languages
- 4. C Program Structure
- 5. Compile and Execute C Program
- 6. Errors in C Program
- 8. C Datatypes
- 9. Operators in C
- Control flow-based C Programs
- Demystifying Bit Masking: Unlocking the Power of Bitwise Operators in C-Language with best Examples.
- 18. Fundamentals of C Functions
- Show Remaining Articles (3)Collapse Articles
Java
AI ML
FAQs
- A Program to find Tokens in C code?
- What are the Common Coding Mistakes.
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
Python Interview Questions
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
18. Fundamentals of C Functions
When we try to write any solution for a complex problem where certain set of statements or code needs to be called multiple times with different set of values, immediately a thought comes to mind that is there any way to call these set of statements as a single task, which may further be called in different ways and different places.
The solution to your thought process can be handled in C by using the functions as single entity where multiple tasks are arranged in way to produce a desired output. But before going into details of the fundamentals of C functions, let us have a brief description about monolithic and Modular Programming.
Monolithic Vs Modular Programming
- Monolithic Programming indicates the program which contains a single function for the large program.
- Modular programming helps the programmer to divide the whole program into different Units and each Unit is separately developed and tested. Then the linker will link all these Units to form the complete program.
- Monolithic programming does not divide the program and operates as a single thread of execution. As the program size increases, it becomes inconvenient and difficult to maintain.
Disadvantages of monolithic programming:
- It is difficult to check errors in large programs.
- Difficult to maintain.
- Code can be specific to a particular problem. i.e. it cannot be reused.
Advantage of modular programming:
- Modular programs are easier to code and debug.
- Reduces the programming size.
- Code can be reused in other programs.
- Problem can be isolated to specific Unit so easier to find the error and correct it.
Introduction to C Function
A function is a group of statements that together perform a task. Every C program has at least one function, which is main (), and all the most trivial programs can define additional functions. Following points will help you to understand functions in c programming language.
- A large program can be divided into the smaller building blocks known as function.
- It contains the set of programming statements enclosed by { …. }.
- A function can be called multiple times to provide reusability and modularity to the C program.
- In other words, we can say that the collection of functions creates a program.
- The function is also known asprocedure orsubroutine in other programming languages.
Advantages of functions
- Functions help in code reusability.
- Functions can be called any number of times in a program and from any place in a program.
- We can track a large C program easily when it is divided into multiple functions.
Types of Functions
Functions in c programming are divided into two different types: 1. Library defined functions and 2. User defined functions.
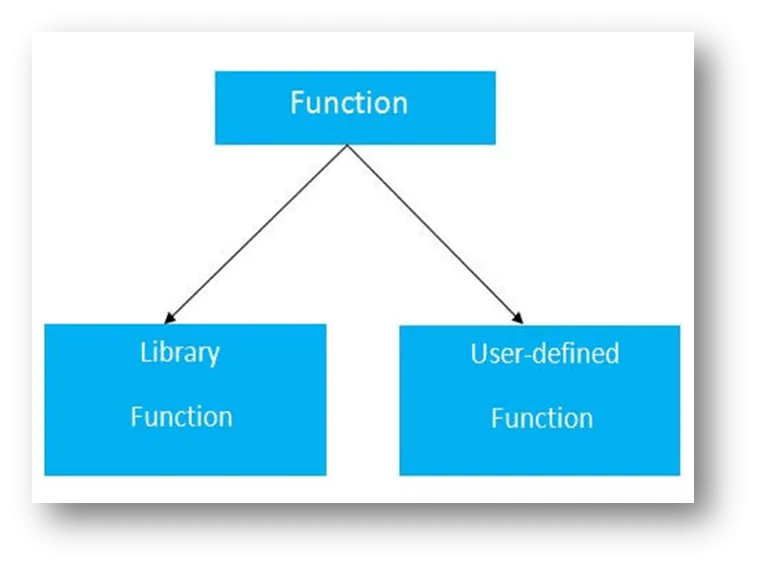
1.Library Functions:
Library functions, like scanf(), printf(), gets(), puts(), ceil(), floor(), etc., are declared in C header files. They are essential for common tasks in C programming, offering a broad range of functionalities that can be extensively accessed and utilized in various programs. These functions are pre-defined in a header file, allowing direct usage in the program without the need for redefinition. This efficiency streamlines the coding process and saves time for the programmer.
2.User-defined functions:
User defined functions are the functions which are created by the C programmer, so that he/she can use it many times. These functions allow for better organization of code, easier debugging, and the ability to reuse code across different parts of a program. By defining functions, programmers can encapsulate a set of instructions into a single unit, making the code more modular and easier to understand. This, in turn, leads to more efficient and maintainable code.
In C programming, no two functions have the same function name and same function declaration. That means no two functions in C have the same function prototype.
The major advantage of User defined function is that it reduces the complexity of a big program and optimizes the code.
Parts of C functions
- a. Function Prototype or Function Declaration
- b. Function Definition.
- Actual Arguments
- c. Calling a function.
- Formal Arguments
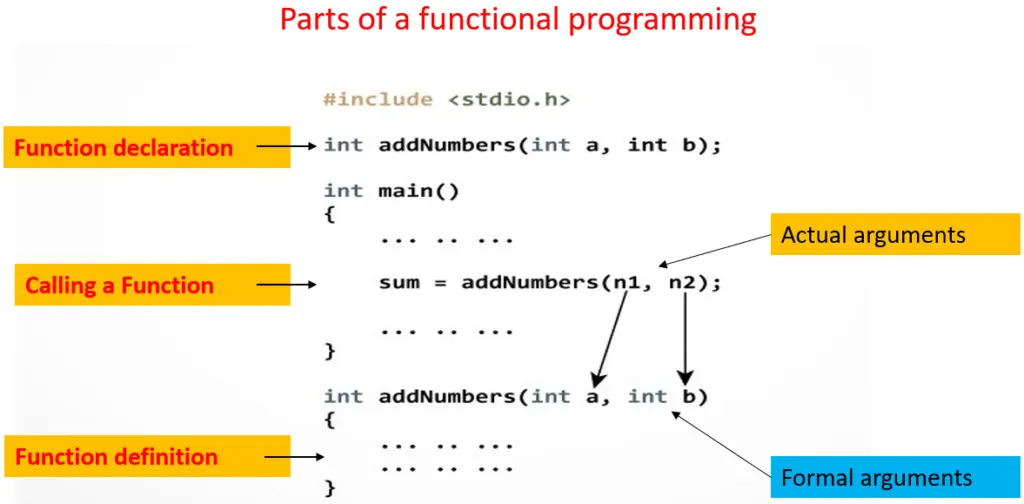
a. Function Declaration OR Function Prototype
- It is also known as function prototype.
- It informs the computer about the three things:
- Name of the function,
- Number and type of arguments received by the function.
- Type of value return by the function
Syntax:
return_type function_name (datatype1 argument1 , datatype2 argument2, ........ );
OR
return_type function_name (datatype1, datatype2, ....... );
Calling function need information about called function. If called function is place before calling function, then the declaration is not needed.
//Example
int addNumbers(int a, int b);
From the above figure of parts of functional programming int addNumbers(int a, int b); is a function prototype.
Actual Arguments:
- a. Arguments which are mentioned in the function call are known as actual arguments.
- b. These are the values which are used at the time of calling a function.
Formal Arguments:
- a) Arguments which are mentioned in function definition are called dummy or formal argument.
- b) These arguments are used to just hold the value that is sent by calling function.
- c) Formal arguments are like other local variables of the function which are created when function call starts and destroyed when end function.
Basic difference between formal and local argument are:
• Formal arguments are declared within the ( ) whereas local variables are declared at beginning.
• Formal arguments are automatically initialized when a value of actual argument is passed.
• Where other local variables are assigned variable through the statement inside the function body.
b. Function Definition:
- It contains the actual statements which are to be executed.
- It is the most important aspect to which the control comes when the function is called.
- Here, we must notice that only one value can be returned from the function.
Syntax:
return type function_name (datatype argumentList) // morethan one aggument should be sapareted by comma
{
local variable;
statements ;
return (expression);
} //end function definition
Function definition can be placed anywhere in the program but generally placed after the main function. Local variable declared inside the function is local to that function. It cannot be used anywhere in the program and its existence is only within the function. Function definition cannot be nested. Return type denote the type of value that function will return and return type is optional if omitted it is assumed to be integer by default.
c. Function call
This is the section of a functional programming where inside any function, another function is being used.
Syntax:
function_name (argument_list);
or
variable = function_name (argument_list);
For example, a function named printf() defined inside head file stdio.h is called inside definition of function main().
//Example1:
#include<stdio.h>
int main()
{
prntf("hello world"); // calling a function
return 0;
}
// Example2:
void myFun ( int , int ); // function declaration
//=====================================================================
int main ( )
{
int a,b;
a= 2;
b=3;
myFun( a, b); // calling a function
return 0;
}
//=====================================================================
void myFun ( int x , int y) // function
{
int s ;
sum = x+y;
printf (“sum = %d” , s ) ;
}
Function Categories
There are four main categories of the functions these are as follows:
- Function with no arguments and no return values.
- Function with no arguments and a return value.
- Function with arguments and no return values.
- Function with arguments and return values.
i. Function with no arguments and no return values
Syntax:
void funct (void);
int main ( )
{
funct ( );
return 0;
}
//=====================================================================
void funct ( void );
{
}
Example: Find factorial of a given number ( no argument, no return )
// type 1: no argument no return
void findFact(void); // function prototype declaration
int main()
{
findFact(); // calling a function
return 0;
}// end main
//=====================================================================
void findFact() // function definition
{
int num,i;
long long int fact=1;
printf("\n Enter any number ");
scanf("%d",&num);
for(i=1;i<=num;++i)
fact=fact*i;
printf("\n fact of num=%d is =%lld", num, fact);
}// end findFact
ii. function with no arguments and with return value
Syntax
return_type function (void);
main ( )
{
datatype var= function ( );
}
//=====================================================================
return_type function ( void );
{
}
Example: Find factorial of a given number (no argument, with return)
//type 2: no argument with return
long long findFact();
int main()
{
long long fct;
fct=findFact();
printf("\n factorial is = %lld",fct);
return 0;
}// end main
//=====================================================================
long long findFact()
{
int num, i;
long long int fact=1;
printf("\n Enter any number ");
scanf("%d",&num);
for(i=1;i<=num;++i)
fact=fact*i;
return fact;
}// end find fact
iii. function with arguments and with no return value
Syntax
void function (datatype arg_list);
main ( )
{
function ( ary_list);
}
//=====================================================================
void function ( datatype ary_list );
{
}
Example: Find factorial of a given number (with argument, with no return)
// type 3: with argument no return
void findFact(int n);
int main()
{
int num;
printf("\n Enter any number ");
scanf("%d",&num);
findFact(num);
return 0;
}// end main
//=====================================================================
void findFact(int n)
{ int i;
long long fact=1;
for(i=1;i<=n;++i)
fact=fact*i;
printf("\n factorial is = %lld",fact);
}// end findFact
iv. function with arguments and with return value
Syntax
return_type function (datatype arg_list);
main ( )
{
datatype var= function ( ary_list);
}
//=====================================================================
void function ( datatype ary_list );
{
}
// type 4: with argument with return
long long findFact(int n);
int main()
{
int num;
long long fct;
printf("\n Enter any number ");
scanf("%d",&num);
fct=findFact(num);
printf("\n factorial is = %lld",fct);
return 0;
}// end main
//========================================================================
long long findFact(int n)
{ int i;
long long fact=1;
for(i=1;i<=n;++i)
fact=fact*i;
return fact;
}// end findFact
Conclusion
Functions in C programing is a utility to use/create library defined / user defined snippet of code which can be used in many ways. The best thing about the function is that they can be treated as “Write Once, Use Many” concept. Functions are classified as two types, one which are already comes with C compiler called Library Functions and another, which are coded by the programmer as per the need of the problem domain. In case of user defined function programmer has to do three things, a. declare b. define and c. call a function. The functions may be of anyone type of its FOUR categories, i. function with no return, no argument. ii. function with return and no argument. iii. Function with no return, with argument(s), and iv. Function with return and with argument(s).