Place Your Query
C Programming
- Control flow-based C Programs
- Enjoy Conditional Programming in C using If...else and switch statement.
- Good, Now write a C program to display "Hello World" on the screen.
- Write a C program to display Your Name, Address and City in different lines.
- C program to find sum of n numbers
- Write a C program For Addition of two numbers using Function.
- Write a Program to Find Simple Interest and Compound Interest.
- Write a Program to Convert Celsius To Fahrenheit and Vice Versa.
- Write a Program to Find Area and Perimeter of Circle, Triangle, Rectangle and Square.
- Write a Program to Swap Two Numbers Using Temporary Variables and Without Using Temporary Variables
- Write a C Program to Design a Simple Menu Driven Calculator
- Simple Expression Based C Programs
- 7. Components of C language
- 1. Introduction to C Programming Language
- 10. Operator Precedence and Associativity
- 11. Comments in C Programming
- 14. Fundamental Control Structure Statements in C [Part-1]
- 15. Fundamental Control Structure Statements in C [Part-2]
- 16. Looping Statements [Fundamental Control Structure Statements in C. #Part-3]
- 17. Keyword break, continue, return and exit [Fundamental Control Structure Statements in C. #Part-4]
- 2. Computer Languages
- 3. Interpreters vs Compilers vs Assemblers in programming languages
- 4. C Program Structure
- 5. Compile and Execute C Program
- 6. Errors in C Program
- 8. C Datatypes
- 9. Operators in C
- Control flow-based C Programs
- Demystifying Bit Masking: Unlocking the Power of Bitwise Operators in C-Language with best Examples.
- 18. Fundamentals of C Functions
- Show Remaining Articles (3)Collapse Articles
Java
AI ML
FAQs
- A Program to find Tokens in C code?
- What are the Common Coding Mistakes.
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
Python Interview Questions
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
Easy Learning, Python QAs for Beginner’s to Pro Part-1
Embarking on the journey of programming can be both thrilling and daunting, especially when diving into a language as versatile as Python. “Learn Python Basics with Questions: A Beginner’s to Pro Approach” is designed to ease you into the world of coding with a gentle, inquiry-based learning method. This approach not only introduces you to the fundamental concepts of Python but also strengthens your understanding through a series of thought-provoking questions.
Whether you’re aspiring to become a software developer, or simply curious about coding, this beginner-friendly guide will help you grasp the essentials of Python. By tackling questions that range from simple syntax to more complex programming logic, you’ll build a solid foundation and develop problem-solving skills that are crucial for any budding programmer.
So, let’s unlock your potential by getting ready for this exciting path to coding proficiency, where every question is a steppingstone to mastering Python basics!
Quick Access
Why is Python called as dynamic and strongly typed language?
Python is called a dynamic and strongly typed language due to its unique combination of features.
Firstly, Python is dynamically typed, which means that the type of a variable is determined at runtime, rather than at compile time. This is in contrast to statically typed languages like C++, where the type of a variable must be explicitly declared and cannot be changed during the execution of the program.
In Python, you can assign a value of any type to a variable, and the type of the variable will be determined by the type of the value you assign to it.
For example, consider the following Python code:
x = 4
print(type(x))
x = "Hello, world"
print(type(x))
In this code, the variable x is first assigned an integer value of.
Then, it is assigned a string value of “Hello, world”. The type() function is used to print the type of the variable x at each step.
In a statically typed language like C++, this code would not be allowed because the type of the variable x would be fixed as an integer.
However, in Python, the type of the variable x is determined dynamically at runtime, and the code runs without any errors. This is because Python is a dynamically typed language.
On the other hand, Python is also a strongly typed language, which means that it has strict rules about how variables of different types can be used. In particular, Python does not allow you to perform operations that are not valid for a particular type of variable. For example, consider the following Python code:
x = 4
y = "Hello, world"
print(x + y)
In this code, the variablex
is an integer, and the variabley
is a string.
The+
operator is used to addx
andy
together. However, this code will raise aTypeError
exception, because you cannot add an integer and a string together in Python.
This is an example of Python’s strong typing in action.
In summary, Python is called a dynamic and strongly typed language because it determines the type of a variable at runtime, and because it has strict rules about how variables of different types can be used. These features make Python a flexible and powerful language for a wide range of applications.
How pass statement is different from a comment?
Thepass
statement in Python is a placeholder statement that does nothing when executed.
It is used when the syntax of the language requires a statement, but there is no code to be executed.
On the other hand, a comment in Python is a piece of text that is ignored by the interpreter. It is used to provide explanations or notes about the code.
The key difference betweenpass
and a comment is thatpass
is not ignored by the interpreter, while a comment is.
This means that if you usepass
in a place where a statement is required, the program will continue to run without any errors.
However, if you use a comment in a place where a statement is required, the program will raise an error.
For example, consider the following code:
if True:
# This is a comment
pass
In this code, thepass
statement is used inside theif
statement. SinceTrue
is always true, theif
statement is executed, and thepass
statement does nothing. If we remove thepass
statement and leave the comment, the program will raise anIndentationError
because theif
statement requires a statement to be executed. Another difference betweenpass
and a comment is thatpass
can be used in places where a statement is required, but a comment cannot.
For example, consider the following code:
def my_function():
# This is a comment
pass
In this code, the pass
statement is used inside a function definition. Since a function definition requires a statement to follow it, the pass
statement is used to satisfy this requirement. If we remove the pass
statement and leave the comment, the program will raise a SyntaxError
because a function definition requires a statement to follow it.
In summary, the pass
statement is a placeholder statement that does nothing when executed, while a comment is a piece of text that is ignored by the interpreter. The key difference between pass
and a comment is that pass
is not ignored by the interpreter, while a comment is. This means that pass
can be used in places where a statement is required, but a comment cannot.
What are the drawbacks of using the pass statement in python?
The drawbacks of using thepass
statement in Python include:Code Readability: Usingpass
too frequently can make the code harder to read and maintain. Ifpass
statements are used excessively, it can clutter the codebase and make it more challenging for other developers to understand the logic.
1. Producing Unused Code: Thepass
statement can lead to the creation of unused and superfluous code. Whenpass
is used excessively, it may result in code that serves no purpose, making the program less efficient and harder to maintain.
2. Maintenance Challenges: Overusing the pass
statement can make the codebase more challenging to maintain. Unnecessarypass
statements can clutter the code, making it harder to identify the actual logic and potentially introducing errors during maintenance or updates.
3. Hiding Logical Mistakes: Usingpass
to fill in code blocks without actual implementation can sometimes hide logical mistakes or errors in the program. This can lead to issues going unnoticed until later stages of development, making debugging more complex.
4. Reduced Code Clarity: Excessive use ofpass
can reduce the clarity of the code. Whenpass
statements are scattered throughout the codebase, it may be difficult for developers to distinguish between actual logic and temporary placeholders, leading to confusion and potential errors.
Finally, One can say that while thepass
statement is a useful tool for creating empty or incomplete code blocks, it should be used judiciously to avoid the drawbacks associated with its overuse. Properly balancing the use ofpass
with other coding practices can help maintain code readability, efficiency, and ease of maintenance in Python programs.
What is the programming cycle for python?
The Python programming cycle is a series of steps that developers follow to create, test, and maintain Python programs. The steps in the Python programming cycle include:
1. Identifying the problem and starting to build the solution: This step involves understanding the problem you want to solve and starting to build a solution. This may involve sketching out a plan, creating a design document, or writing some initial code.
2. Writing the source code: Once you have a plan in place, you can start writing the source code for your solution. This may involve creating functions, classes, and modules, and writing the logic for your program.
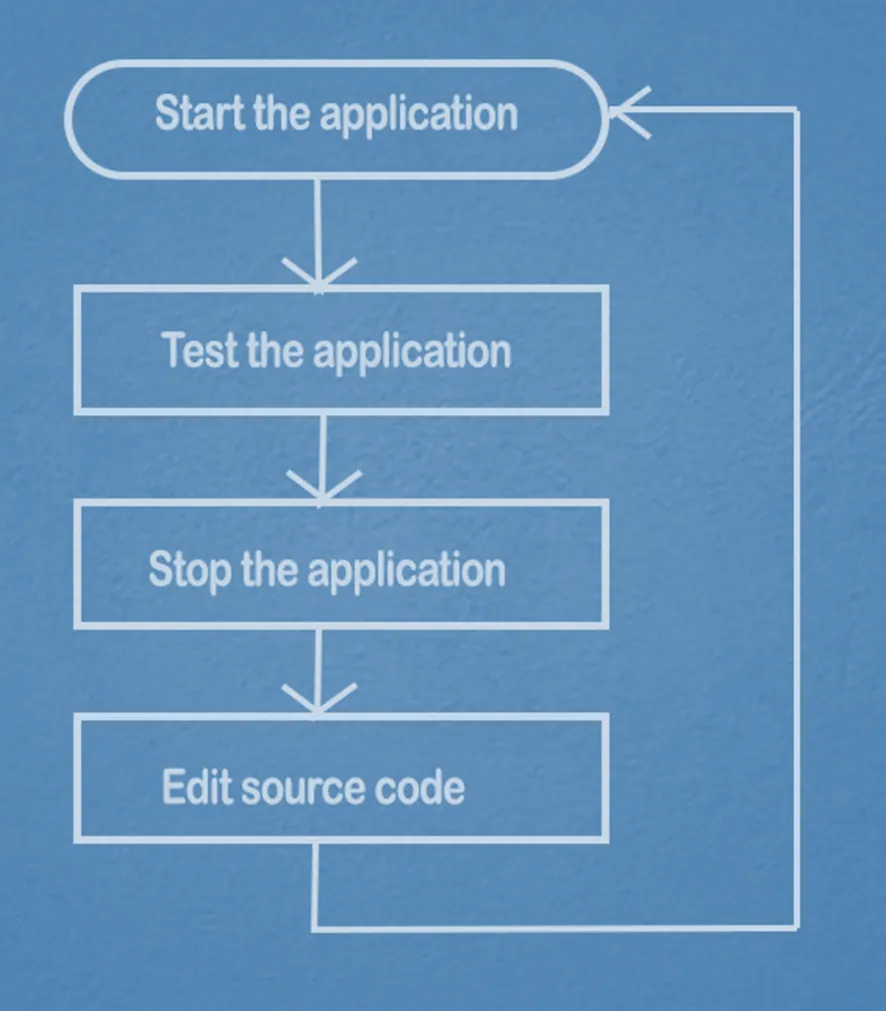
3. Testing the code: After you have written the source code, you should test it to ensure that it works correctly. This involves running the program with different inputs and checking the outputs.
4. Debugging: If you find any syntax or logical errors during testing, you will need to debug the code to fix them. This involves identifying the cause of the error and modifying the code to correct it.
5. Updating the program: After the program is working correctly, you may need to update it from time to time to add new features or fix bugs.
The Python programming cycle is comparatively shorter and easier than the life cycles of traditional programming languages.
Python is an interpreted language, which means that it executes the program line by line and stops at the first place it finds an error. This makes it easy to debug code, as you can see the exact line where the error occurred.
Additionally, Python programs can import modules at runtime, which means that you can easily add new functionality to your program without recompiling it. Python IDEs such as PyCharm, Spyder, PyDev, and IDLE can help streamline the programming cycle by providing tools for editing, testing, and debugging code. These IDEs can help automate the process of writing and testing code, making it easier to create high-quality Python programs.
What is Type Conversion in Python?
Type conversion in Python refers to the process of changing a value from one data type to another. This is often done to perform specific operations that require a certain data type, or to ensure data compatibility.
There are two types of type conversion in Python: implicit and explicit.
- Implicit Type Conversion:
- Also known as type coercion, it is the automatic conversion of data from one data type to another by the programming language without explicit request from the programmer.
- For example, when an integer and a float are added, the integer is automatically converted to a float to perform the operation.
- Explicit Type Conversion:
- Also known as type casting, it is the deliberate process of changing a value from one data type to another by specifying the data type explicitly.
- This is done using built-in functions like
int()
,float()
,str()
,bool()
, andcomplex()
.
- Built-in Functions for Type Conversion:
- Python provides several built-in functions for explicit type conversion:
int()
: Converts a value to an integer.float()
: Converts a value to a float.str()
: Converts a value to a string.bool()
: Converts a value to a boolean.complex()
: Converts a value to a complex number.
- Python provides several built-in functions for explicit type conversion:
- Data Loss:
- Type conversion can result in data loss, especially when converting from a larger data type to a smaller one.
- For example, converting a float to an integer will result in the loss of the decimal part of the number.
- Importance:
- Understanding type conversion is crucial for manipulating data and ensuring compatibility in Python programs.
- It allows for flexibility in handling different data types and performing operations that require specific data types.
Summary of difference between Implicit and Explicit Conversions
By following four points, you can effectively grasp the concept of type conversion in Python and utilize it to manipulate data efficiently in your programs.
Feature | Implicit Conversion | Explicit Conversion |
---|---|---|
Initiation | Automatic by Python | Manual by the programmer |
Data Loss | No data loss | Possible data loss |
Common Use Case | Arithmetic operations | When specific type is required |
Conversion Functions | None (automatic) | int() ,float() ,str() , etc. |
What is the syntax/rule for swapping two numbers in python?
The syntax for swapping two numbers in Python is not a single line of code, but a concept that can be implemented in multiple ways. The most common methods for swapping two numbers in Python are:
- Using a temporary variable: In this method, a temporary variable is used to store the value of one variable, and then the values are swapped. The syntax for this method is:
temp = x
x = y
y = temp
2. Using XOR operator: This method uses the XOR operator to swap the values of two variables. The syntax for this method is:
x = x ^ y
y = x ^ y
x = x ^ y
3. Using arithmetic operators: This method uses arithmetic operators to swap the values of two variables. The syntax for this method is:
x = x + y
y = x - y
x = x - y
4. Using bitwise operators: This method uses bitwise operators to swap the values of two variables. The syntax for this method is:
x = (x & y) | (x ^ y)
y = (x ^ y) & ~(x & y)
x = x ^ y
5. Using comma operator: This method uses the comma operator to swap the values of two variables. The syntax for this method is:
x, y = y, x
These are some of the common methods for swapping two numbers in Python. The choice of method depends on the specific requirements and constraints of the problem at hand.
In this program:
- The user is prompted to enter two numbers.
- The numbers are displayed before swapping.
- The numbers are then swapped without using a temporary variable by performing arithmetic operations.
- Finally, the swapped numbers are displayed to the user.
You can run this program in a Python environment, and it will swap the two numbers entered by the user without using any intermediate variables.
# Prompt the user to enter two numbers
num1 = float(input("Enter the first number: "))
num2 = float(input("Enter the second number: "))
# Display the numbers before swapping
print("Before swapping:")
print("First number:", num1)
print("Second number:", num2)
# Swap the numbers without using a temporary variable
num1 = num1 + num2
num2 = num1 - num2
num1 = num1 - num2
# Display the numbers after swapping
print("\nAfter swapping:")
print("First number:", num1)
print("Second number:", num2)
Define memory management in python.
Memory management in Python refers to the way the Python programming language handles the allocation and deallocation of memory resources during program execution. Python’s memory management system is responsible for efficiently managing memory usage, allocating memory when needed by the program, and releasing memory that is no longer in use. Key aspects of memory management in Python include:
Memory Management: From Hardware to Software:
- Memory management is the process of reading and writing data. The memory manager determines where an application’s data should reside.
- Since memory is finite (like the pages in our book analogy), the manager allocates free space to applications.
- When data is no longer needed, it can be deleted or freed
- Automatic Memory Management: Python automatically handles memory allocation and deallocation, relieving developers from the manual task of managing memory. This automatic memory management is achieved through techniques like reference counting and garbage collection.
- Reference Counting: Python uses reference counting as a memory management technique. Each object in Python has a reference count associated with it, which tracks the number of references to that object. When the reference count of an object reaches zero, the object is deleted from memory.
- Garbage Collection: Python’s garbage collector is responsible for freeing up memory that is no longer in use. The garbage collector periodically scans the memory to identify objects with a reference count of zero and deallocates them. This process helps prevent memory leaks and ensures efficient memory usage.
- Heap Memory Allocation: Python uses a private heap to store program objects and data structures. Memory allocation in Python involves allocating memory on the heap for objects created during program execution. Python uses different memory allocation strategies based on the type and size of the objects being created.
- Efficient Memory Management: Python’s memory management system is designed to be simple, efficient, and automatic. It optimizes memory usage by minimizing memory fragmentation and ensuring that memory is released when no longer needed.
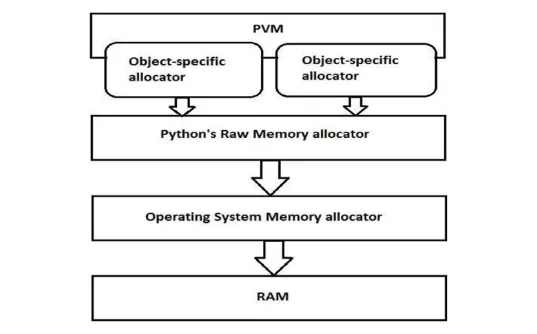
Overall, memory management in Python plays a crucial role in ensuring that programs run efficiently, manage memory resources effectively, and prevent issues like memory leaks. By abstracting away the complexities of memory management, Python allows developers to focus on writing code without the need to manually handle memory allocation and deallocation.
What is python private heap in memory management?
The Python private heap is a memory management mechanism used by the Python interpreter to allocate and manage memory for Python objects and data structures. The Python memory is primarily managed by the Python private heap space, where all Python objects and data structures are located. In detail one can observe private heap as:
- The Python private heap is a private memory space managed by the Python interpreter, which is separate from the system’s heap space or the stack space.
- The Python private heap is used to store all the values and objects of Python, while the references to these objects are stored in the stack memory.
- The Python private heap is managed by Python’s built-in garbage collector, which continuously looks for unreferenced objects and deletes them from memory, ensuring good optimization of memory at the cost of the speed of code execution.
- The Python private heap is used to allocate memory for Python objects and other internal buffers on demand, and it is managed by Python’s memory manager, which has different components that deal with various dynamic storage management aspects, such as sharing, segmentation, pre-allocation, or caching1.
- The Python private heap is used to store all the objects and data structures, while its counterpart, the stack space, contains all the references to the objects in the heap space.
- When an object is updated, the new value is written at a new location, and the variable is referenced to the new address, while the old object is deleted from the heap space by the garbage collector as soon as references to the object reach zero.
- The Python private heap is used to store all the values and objects of Python, while the references to these objects are stored in the stack memory, and the garbage collector continuously looks for unreferenced objects and deletes them from memory, ensuring good optimization of memory at the cost of the speed of code execution.
How does python’s private heap differ from the stack memory?
Python’s private heap and stack memory serve different purposes in memory management. Here is a comparison of Python’s private heap and stack memory based on the provided sources:
Python’s Private Heap:
- Function: The private heap in Python is used to store all Python objects and data structures.
- Management: The private heap is managed internally by the Python memory manager, which ensures efficient memory allocation and deallocation.
- Components: The Python memory manager has different components that deal with various dynamic storage management aspects within the private heap.
- Allocation: Memory for Python objects and internal buffers is allocated on demand by the Python memory manager within the private heap.
- Object-Specific Allocators: Object-specific allocators operate on the same heap and implement distinct memory management policies tailored to specific object types.
- Flexibility: The private heap provides flexibility in memory allocation and storage for Python objects and data structures.
- Garbage Collection: The private heap is managed by Python’s built-in garbage collector, which continuously looks for unreferenced objects and deletes them from memory to optimize memory usage.
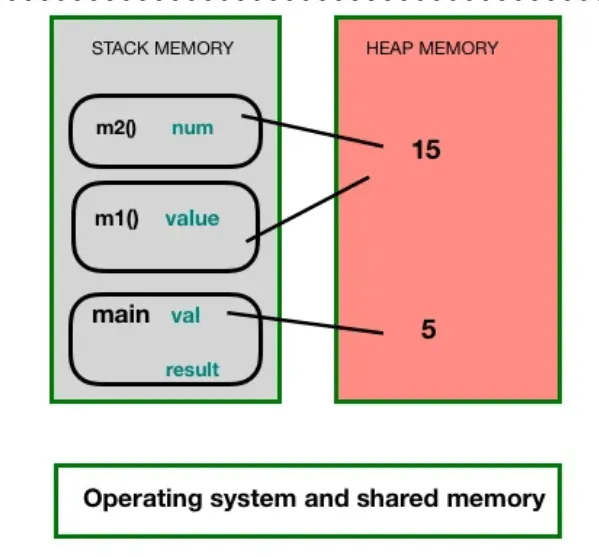
Stack Memory:
- Function: The stack memory in Python stores references to objects on the heap, while the actual data of the objects is stored in the heap memory.
- Allocation: Stack memory allocation happens in contiguous blocks of memory and is used for storing local variables and function call information.
- Temporary Storage: Stack memory is used for temporary storage of local variables within a function or method call.
- Function Calls: When a function is called, its variables are stored on the stack, and they are removed once the function execution completes.
- Efficiency: Storing primitive data types on the stack is efficient due to their fixed size and simple data structure.
- Reference Storage: The stack stores references to objects on the heap, pointing to the actual data stored in the heap memory.
- Multiple References: Multiple variables can reference the same object on the heap, with the reference stored on the stack.
Finally, Python’s private heap is used to store Python objects and data structures, managed by the Python memory manager, while stack memory stores references to objects on the heap and local variables during function calls. The private heap provides flexibility and efficient memory management, while stack memory is used for temporary storage and function call information.