Place Your Query
C Programming
- Control flow-based C Programs
- Enjoy Conditional Programming in C using If...else and switch statement.
- Good, Now write a C program to display "Hello World" on the screen.
- Write a C program to display Your Name, Address and City in different lines.
- C program to find sum of n numbers
- Write a C program For Addition of two numbers using Function.
- Write a Program to Find Simple Interest and Compound Interest.
- Write a Program to Convert Celsius To Fahrenheit and Vice Versa.
- Write a Program to Find Area and Perimeter of Circle, Triangle, Rectangle and Square.
- Write a Program to Swap Two Numbers Using Temporary Variables and Without Using Temporary Variables
- Write a C Program to Design a Simple Menu Driven Calculator
- Simple Expression Based C Programs
- 7. Components of C language
- 1. Introduction to C Programming Language
- 10. Operator Precedence and Associativity
- 11. Comments in C Programming
- 14. Fundamental Control Structure Statements in C [Part-1]
- 15. Fundamental Control Structure Statements in C [Part-2]
- 16. Looping Statements [Fundamental Control Structure Statements in C. #Part-3]
- 17. Keyword break, continue, return and exit [Fundamental Control Structure Statements in C. #Part-4]
- 2. Computer Languages
- 3. Interpreters vs Compilers vs Assemblers in programming languages
- 4. C Program Structure
- 5. Compile and Execute C Program
- 6. Errors in C Program
- 8. C Datatypes
- 9. Operators in C
- Control flow-based C Programs
- Demystifying Bit Masking: Unlocking the Power of Bitwise Operators in C-Language with best Examples.
- 18. Fundamentals of C Functions
- Show Remaining Articles (3)Collapse Articles
Java
AI ML
FAQs
- A Program to find Tokens in C code?
- What are the Common Coding Mistakes.
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
Python Interview Questions
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
Learning Python basics through questions is a fantastic way to grasp the fundamentals of Python programming. This article delves into String Basics, explaining that Python strings are collections of characters enclosed in single, double, or triple quotes. We’ll explore indexing and slicing, where string indexing begins at 0, and reverse indexing starts from -1. Remember, Python strings are immutable, but you can create new strings by reassigning variables. Internally, characters are stored as binary streams, encoded using ASCII or Unicode. Furthermore, we’ll discuss accessing individual characters, known as string slicing, using square brackets that hold the index or reverse index.
What are Python Strings?
One can understand the python strings will the help of following short description:
- Python string is the collection of the characters surrounded by single quotes, double quotes, or triple quotes.
- The computer does not understand the characters, internally, it stores manipulated character as binary stream.
- Each character is encoded in the ASCII or Unicode character. So we can say that Python strings are also called the collection of Unicode characters.
How to create a String in Python
The following code demonstrates different syntaxes for creating string variables in Python. Here’s a brief explanation of each syntax:
- 1. `str_var=”your string”` – This syntax uses double quotes to define a string variable.
- 2. `str_var=’your string’` – This syntax uses single quotes to define a string variable.
- 3. `str_var=”’your strng”’` – This syntax uses triple single quotes to define a multi-line string variable.
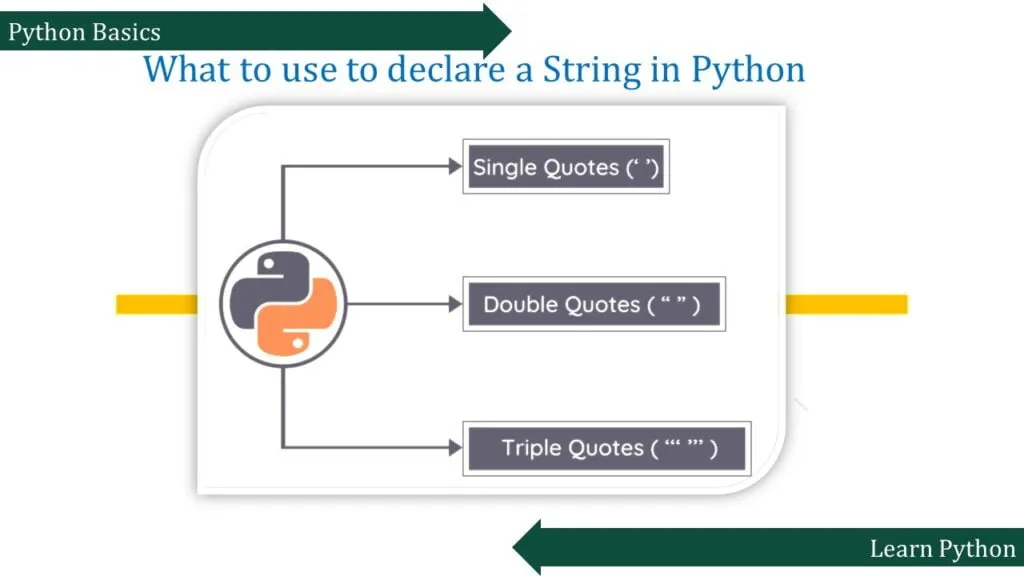
#Syntax:
str_var="your string"
str_var='your string'
str_var='''your strng'''
# Example-1: #Using single quotes
str1 = 'Ezy Learning'
print(str1)
# Example-2: Using double quotes
str2 = "Ezy Learning"
print(str2)
# Example-3: #Using triple quotes
str3 = '''''Python string is the collection
of the characters surrounded
by single quotes,
double quotes, or triple
quotes'''
print(str3)
Output
Ezy Learning
Ezy Learning
''Python string is the collection
of the characters surrounded
by single quotes,
double quotes, or triple
quotes
How indexing and splitting works with Python Strings?
Like other languages, the indexing of the Python strings starts from 0.
For example, The string “HELLO” is indexed as given in the below figure
Like other languages, Python string indexing starts at 0.
For example, the string “HELLO” is indexed as shown below.
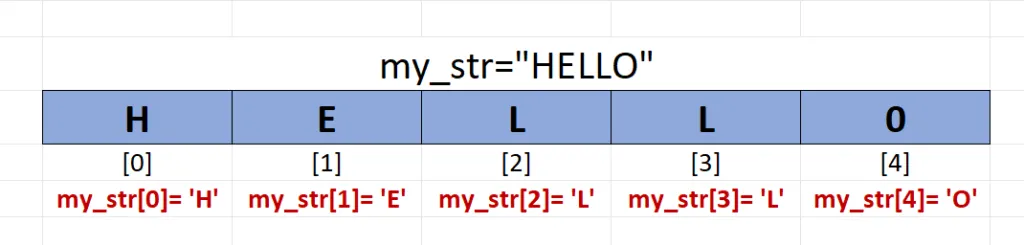
- This means that the first character “H” has an index of 0,
- The second character “E” has an index of 1, and so on.
- One can access individual characters in a string using their index in square brackets.
- For example, to access the first character of the string “HELLO”, you would write
my_string[0]
. This would return the character “H”.
You can also access a range of characters in a string using slicing. To access the first three characters of the string “HELLO”, you would writemy_string[0:3]
. This would return the string “HEL”.
Keep in mind that in the python strings, the upper bound of the slice is exclusive, so the character at index 3 (“L”) is not included in the slice.
# Define a string variable
my_str = 'HELLO'
# Use a try-except block to handle potential index out of range error
try:
# Print each character of the string
print(my_str[0])
print(my_str[1])
print(my_str[2])
print(my_str[3])
print(my_str[4]) # This line will cause an index out of range error
print(my_str[5]) # This line will not be executed due to the error in the previous line
print(my_str[6]) # This line will not be executed due to the error in the previous line
# Catch any exception that occurs and print the error message
except Exception as e:
print(e)
Output
H
E
L
L
O
string index out of range
`except Exception as e:` – This block catches any exception that occurs within the `try` block and assigns it to the variable `e`. 10. `print(e)` – This line prints the exception that occurred, which in this case would be an IndexError due to attempting to access an index that is out of range for the string.
Access Substring
- the slice operator [] is used to access the individual characters of the string.
- However, we can use the : (colon) operator in Python to access the substring from the given string.
## Example
# Given String
str = "EzyLearning.in"
# Start Oth index to end
print(str[0:]) #--> EzyLearning.in
# Starts 1th index to 4th index
print(str[1:5]) #--> zyLe
# Starts 2nd index to 3rd index
print(str[2:4]) #--> yL
# Starts 0th to 2nd index
print(str[:3]) #--> Ezy
#Starts 4th to 6th index
print(str[4:7]) #--> ear
Output
EzyLearning.in
zyLe
yL
Ezy
ear
# apply slicing
print(str[4:4])
## this will give no results.... please share your view for this question in the comment section.
## this will give no results (i.e. empty string) .... please share your view for this question in the comment section.
Fail to find the cause, No issues, just read the following:
The selected code `print(str[4:4])` attempts to print a substring of the variable `str` from index 4 to index 4. However, this will result in an empty string being printed, as the start and end indices are the same. In Python, when slicing a string, the start index is inclusive, and the end index is exclusive. Therefore, in the python strings, when the start and end indices are the same, an empty string is returned.
Does reverse indexing and slicing based on it works in python?
Yes, Python also supports a reverse index, so negative slicing is possible
- We can do the negative slicing in the string; it starts from the rightmost character,
- which is indicated as -1.
- The second rightmost index indicates -2, and so on.
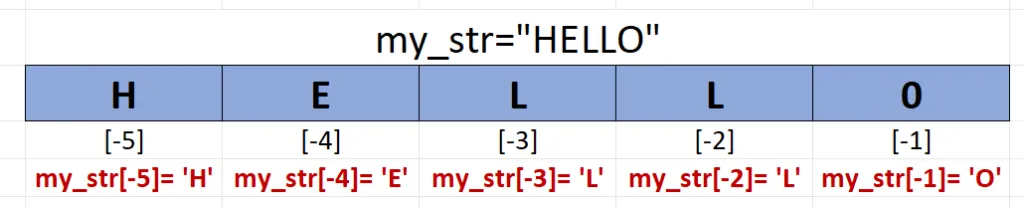
# Accessing the last character of the string
print(str[-1])
# Accessing the third character from the end of the string
print(str[-3])
# Accessing the last two characters of the string
print(str[-2:])
# Accessing a substring from the fourth character from the end to the second character from the end
print(str[-4:-1])
# Accessing a substring from the seventh character from the end to the third character from the end
print(str[-7:-2])
Output
g
n
ng
rnn
Learn
# Reversing the given string
print(str[::-1]) # Print the string in reverse order
print(str[-8:-2]) # Print a substring of the string from index -8 to -3
Output
gnnraeLyzE
yLearn
# This line of code prints a substring of the string 'str' starting from index 4 (exclusive) and ending at index 2 (inclusive) in reverse order.
print(str[4:2:-1])
Output
eL
Explanation:
- ‘str[4 : 2 : -1]` – This uses string slicing to extract a substring from the original string ‘str’.
- The slicing syntax is `[start : stop: step]`. – `4`
- This is the starting index for the substring `2`
- This is the ending index for the substring (exclusive) `-1`
- This is the step value, indicating that the substring should be traversed in reverse order.
- When this code is executed, it will print the substring of ‘str’ starting from index 4 and ending at index 3 (exclusive) in reverse order.
Do you Reassign a String?
a. Strings are immutable in Python.
b. A string can only be replaced with new string since itscontent cannot be partially replaced.
c. Updating the content of the strings is as easy as assigning it to a new string.
d. The string object doesn’t support item assignment i.e., partial updation not allowed but total replacement is allowed
Example -1
# Define a string variable
string = "HELLO"
try:
# Attempt to modify the first character of the string
string[0] = "h"
print(string)
except Exception as e:
# If an exception occurs, print the error message
print(e)
Output:
'str' object does not support item assignment
Explanation:
- The above-mentioned code attempts to modify the first character of the string “HELLO” from ‘H’ to ‘h’, and then prints the modified string.
- However, since strings in Python are immutable (cannot be changed), this will result in an error.
- Here’s a step-by-step explanation of the selected code:
- 1.
`str = "HELLO"`
– This line initializes a string variable `str` with the value “HELLO”. - 2.
`try:`
– This keyword marks the beginning of a block of code where an exception might occur. - 3.
`str[0] = "h"`
– This line attempts to modify the first character of the string `str` from ‘H’ to ‘h’. This operation will raise an error because strings in Python are immutable. - 4. `
print(str)`
- 5.
`except Exception as e:`
– This block catches any exception that occurs within the `try` block and assigns it to the variable `e`. - 6.
`print(e)`
– This line prints the exception that occurred, which in this case will be “TypeError: ‘str’ object does not support item assignment”.
- 1.
- However, the string str can be assigned completely to a new content as specified in the following example.
Example 2
# Set the value of the variable str to "HELLO"
str = "HELLO"
# Print the value of str
print(str)
# Set the value of the variable str to "hello"
str = "hello"
# Print the value of str
print(str)
# Output:
HELLO
hello
Explanation:
The above code snippet contains two lines of code:
- 1. `tr = “HELLO”` – This line creates a new variable `tr` and assigns the string “HELLO” to it.
- 2. `print(str)` – This line prints the value of the variable `str`. However, it seems that there is a mistake in this line. The variable `str` has not been defined in this snippet, so this line will result in an error.
- 3. `str = “hello”` – This line creates a new variable `str` and assigns the string “hello” to it.
- 4. `print(str)` – This line prints the value of the variable `str`, which is “hello”.
What are your views on Deleting the String?
a. As we know that strings are immutable. We cannot delete or remove the characters from the string.
b. But we can delete the entire string using the del keyword.
Example
## partial deletion not allowed, will give and error : TypeError: 'str' object doesn't support item deletion
str = " EzyLearning " ## Define a string variable
try:
del str[1] ## Try to delete a character at index 1, which is not allowed for strings
except Exception as e:
print(e) ## Print the exception message if deletion is not allowed
# Output:
'str' object doesn't support item deletion
Now we are trying to delete an entire string.
The given code initializes a variable `str1` with the value “EzyLearning”, then deletes the variable using the `del` keyword and attempts to print the value of `str1`.
However, since the variable has been deleted, an error will occur when trying to print `str1`.
# The selected code attempts to delete the variable 'str1' and then print its value, which should result in an error since the variable has been deleted.
str1 = "EzyLearning" # Create a new string variable
del str1 # Delete the variable 'str1'
# Attempt to print the value of the deleted variable, which should raise an exception
try:
print(str1)
except Exception as e:
print(e) # Print the exception message if an exception occurs
# Output:
name 'str1' is not defined