Place Your Query
-
C Programming
-
- Control flow-based C Programs
- Enjoy Conditional Programming in C using If...else and switch statement.
- Good, Now write a C program to display "Hello World" on the screen.
- Write a C program to display Your Name, Address and City in different lines.
- C program to find sum of n numbers
- Write a C program For Addition of two numbers using Function.
- Write a Program to Find Simple Interest and Compound Interest.
- Write a Program to Convert Celsius To Fahrenheit and Vice Versa.
- Write a Program to Find Area and Perimeter of Circle, Triangle, Rectangle and Square.
- Write a Program to Swap Two Numbers Using Temporary Variables and Without Using Temporary Variables
- Write a C Program to Design a Simple Menu Driven Calculator
- Simple Expression Based C Programs
-
- 7. Components of C language
- 1. Introduction to C Programming Language
- 10. Operator Precedence and Associativity
- 11. Comments in C Programming
- 14. Fundamental Control Structure Statements in C [Part-1]
- 15. Fundamental Control Structure Statements in C [Part-2]
- 16. Looping Statements [Fundamental Control Structure Statements in C. #Part-3]
- 17. Keyword break, continue, return and exit [Fundamental Control Structure Statements in C. #Part-4]
- 2. Computer Languages
- 3. Interpreters vs Compilers vs Assemblers in programming languages
- 4. C Program Structure
- 5. Compile and Execute C Program
- 6. Errors in C Program
- 8. C Datatypes
- 9. Operators in C
- Control flow-based C Programs
- Demystifying Bit Masking: Unlocking the Power of Bitwise Operators in C-Language with best Examples.
- 18. Fundamentals of C Functions
- Show Remaining Articles (3)Collapse Articles
-
-
Java
-
AI ML
-
FAQs
- A Program to find Tokens in C code?
- What are the Common Coding Mistakes.
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
-
Python Interview Questions
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
16. Looping Statements [Fundamental Control Structure Statements in C. #Part-3]
Program Loops and IterationA loop statement allows us to execute a statement or group of statements multiple times and following is the general from of a loop statement in most of the programming languages:
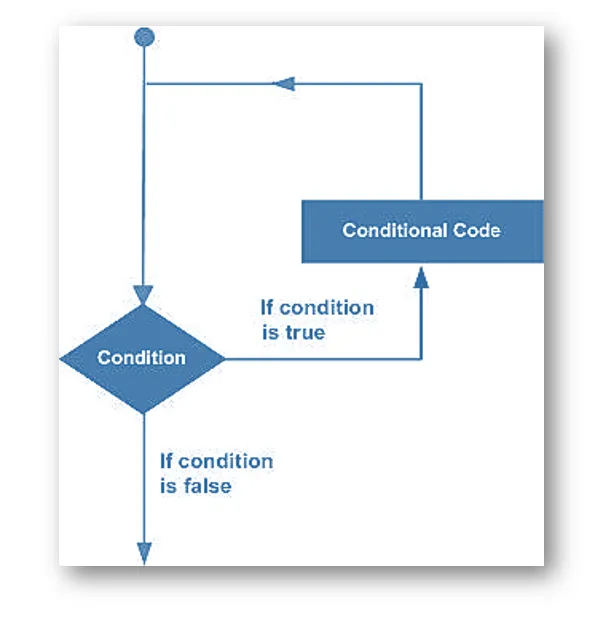
Loop-Control-Type
a. Counter control: Number of iteration time is known.
b. Sentinel Control (Infinite Loop): Number of iterations is not known, but they are controlled by special variable value like-
- “end-of-life”,
- value between -1 to 9,
- until ‘E’ is not pressed, etc.
Advantages
- It provides code reusability.
- Using loops, we do not need to write the same code again and again.
- Using loops, we can traverse over the elements of data structures (array or linked lists).
Types of C Loops
There are three types of loops in C language:
- 1. do… while () Loop
- 2. while () Loop
- 3. for () Loop
Loop Control Structure
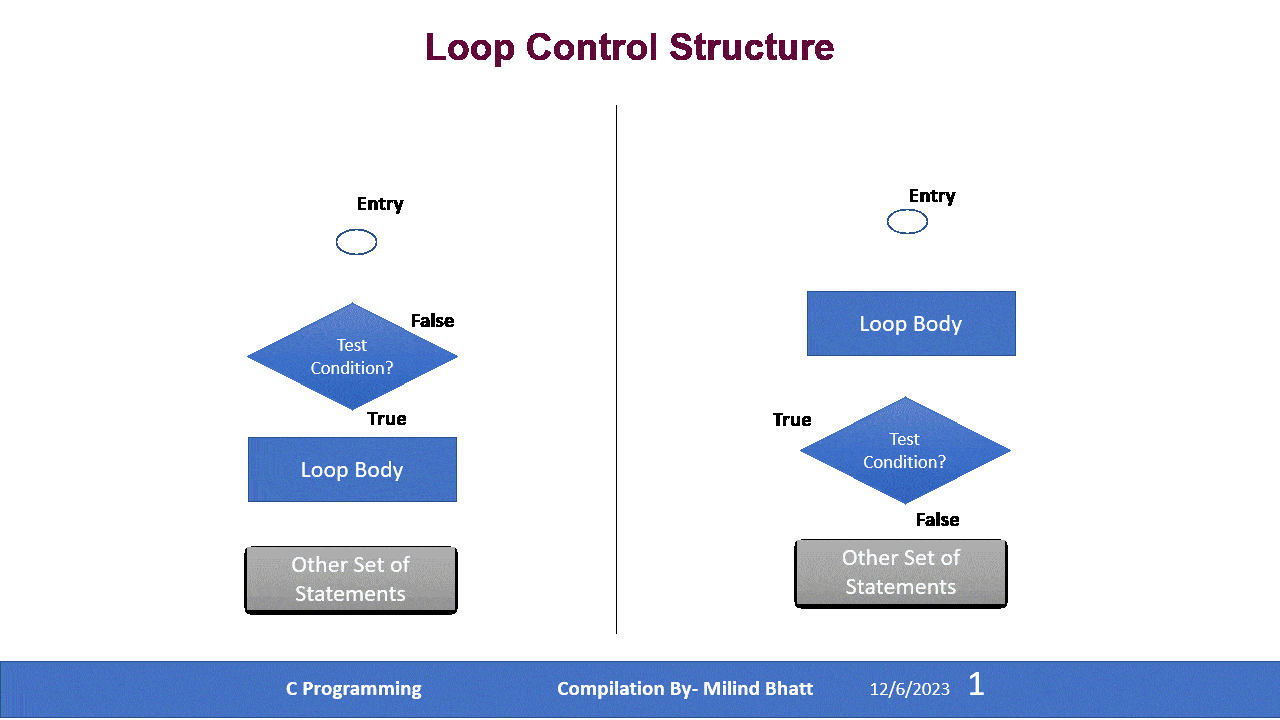
1. While () Loop
A while loop statement in C programming language repeatedly executes a target statement(s) as long as a given condition remains true.
Syntax:
while(condition)
{
statement(s);
}
Here statement(s) may be a single statement or a block of statements. The condition may be any expression, and true is any nonzero value. The loop iterates while the condition is true. When the condition becomes false, program control passes to the line immediately following the loop.
Flow Diagram:
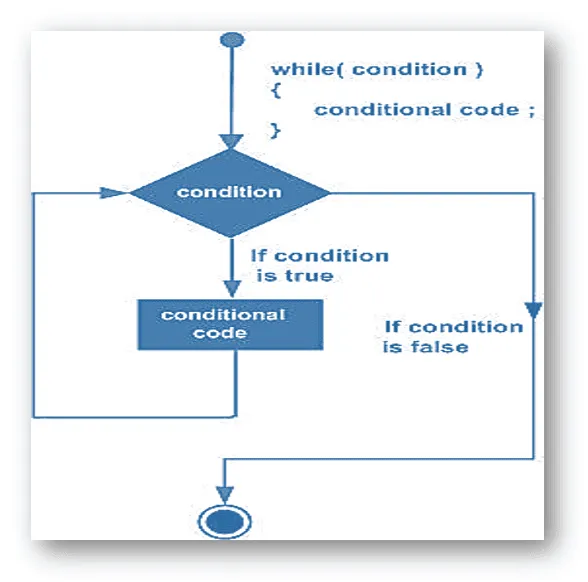
Example-1
// Sum of first 30 numbers
#include<stdio.h>
void main()
{ int sum=0, n=1;
while(n<=30)
{sum = sum + n;
n++;
}// end while
printf("\n sum of 1 to 30 =%d", sum);
}// end main
Output:
sum of 1 to 30 =465
Example-2
#include <stdio.h>
main ()
{
int a = 10;
while( a < 15 )
{
printf("value of a: %d\n", a);
a++;
}
}// end main
When the above code is compiled and executed, it produces following result: value of a: 10
Output:
value of a: 11
value of a: 12
value of a: 13
value of a: 14
value of a: 15
Unlike for and while loops, which test the loop condition at the top of the loop, the do…while loop in C programming language checks its condition at the bottom of the loop. A do…while loop is similar to a while loop, except that a do…while loop is guaranteed to execute at least one time.
2. do…While () Loop
Here all the loop-block statements undergo for their first execution and if the condition is true, the flow of control jumps back up to do, and the statement(s) in the loop execute again. This process repeats until the given condition becomes false. Also do…while () loop ensure that your loop-body (i.e. loop-block) statements will execute at least once, irrespective of the test-condition.
Syntax:
do
{
//Statement(s);
} while ( condition );
Flow Diagram:
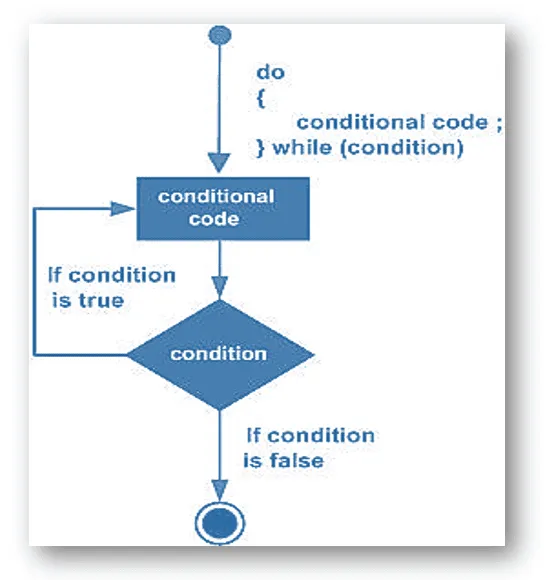
Example-1
#include <stdio.h>
main ()
{ int a = 10;
do
{
printf("value of a: %d\n", a); a = a + 1;
} while ( a < 16 );
}// end main
When the above code is compiled and executed, it produces following result: value of a: 10
Output:
value of a: 11
value of a: 12
value of a: 13
value of a: 14
value of a: 15
Example-2
// Sum of first 30 numbers
#include<stdio.h>
void main()
{ int sum=0, n=1;
do
{sum = sum + n;
n++;
} while(n<=30); // end while
printf("\n sum of 1 to 30 =%d", sum);
}// end main
Output:
sum of 1 to 30 =465
Difference between while() and do…while() statements.
BASIS FOR COMPARISON | WHILE | DO-WHILE |
General Form | while ( condition) { statements; //body of loop} | do{ statements; // body of loop. } while( Condition ); |
Controlling Condition | In ‘while’ loop the controlling condition appears at the start of the loop. | In ‘do-while’ loop the controlling condition appears at the end of the loop. |
Iterations | The iterations do not occur if, the condition at the first iteration, appears false. | The iteration occurs at least once even if the condition is false at the first iteration. |
Alternate name | Entry-controlled loop | Exit-controlled loop |
Semi-colon | Not used | Used at the end of the loop |
3. For () loops
A for () loop is a repetition control structure that allows you to efficiently write a loop that needs to execute a specific number of times.
Syntax:
for ( initialization ; condition; increment )
{
statement(s);
}
Flowchart
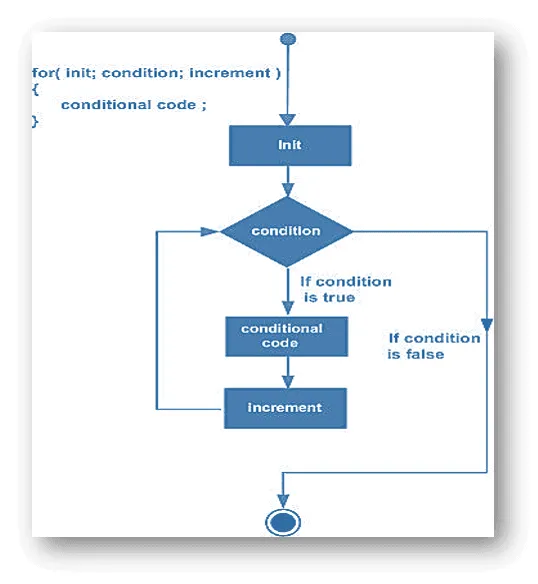
#include <stdio.h>
main ()
{
for( int num = 15; a < 20; num = num + 1 )
{
printf("value of num: %d\n", num);
}
}
Output:
value of num: 15
value of num: 16
value of num: 17
value of num: 18
value of num: 19