Place Your Query
C Programming
- Control flow-based C Programs
- Enjoy Conditional Programming in C using If...else and switch statement.
- Good, Now write a C program to display "Hello World" on the screen.
- Write a C program to display Your Name, Address and City in different lines.
- C program to find sum of n numbers
- Write a C program For Addition of two numbers using Function.
- Write a Program to Find Simple Interest and Compound Interest.
- Write a Program to Convert Celsius To Fahrenheit and Vice Versa.
- Write a Program to Find Area and Perimeter of Circle, Triangle, Rectangle and Square.
- Write a Program to Swap Two Numbers Using Temporary Variables and Without Using Temporary Variables
- Write a C Program to Design a Simple Menu Driven Calculator
- Simple Expression Based C Programs
- 7. Components of C language
- 1. Introduction to C Programming Language
- 10. Operator Precedence and Associativity
- 11. Comments in C Programming
- 14. Fundamental Control Structure Statements in C [Part-1]
- 15. Fundamental Control Structure Statements in C [Part-2]
- 16. Looping Statements [Fundamental Control Structure Statements in C. #Part-3]
- 17. Keyword break, continue, return and exit [Fundamental Control Structure Statements in C. #Part-4]
- 2. Computer Languages
- 3. Interpreters vs Compilers vs Assemblers in programming languages
- 4. C Program Structure
- 5. Compile and Execute C Program
- 6. Errors in C Program
- 8. C Datatypes
- 9. Operators in C
- Control flow-based C Programs
- Demystifying Bit Masking: Unlocking the Power of Bitwise Operators in C-Language with best Examples.
- 18. Fundamentals of C Functions
- Show Remaining Articles (3)Collapse Articles
Java
AI ML
FAQs
- A Program to find Tokens in C code?
- What are the Common Coding Mistakes.
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
Python Interview Questions
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
Good, Now write a C program to display “Hello World” on the screen.
In this article, we will learn how to write a C program to display “Hello World” on the screen. This is a simple program that can be used to demonstrate the basic syntax and structure of C language.
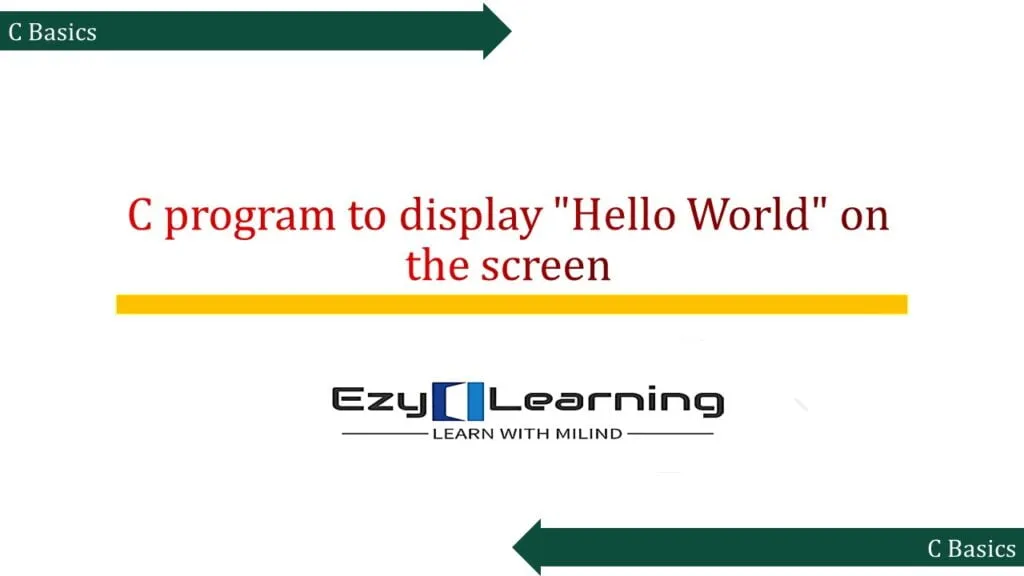
What we need?
To write a C program, we need a text editor and a compiler. A text editor is a software that allows us to write and edit the source code of our program. A compiler is a software that converts the source code into an executable file that can be run by the computer.
The first step is to create a new file in our text editor and save it with a .c extension. For example, we can name our file ezyfirst.c. The .c extension indicates that the file contains C source code.
The second step is to write the source code of our program. A C program consists of one or more functions, which are blocks of code that perform specific tasks. The main function is the starting point of the program, where the execution begins.
To display “Hello World” on the screen, we need to use the printf function, which is a built-in function that prints a formatted string to the standard output device, usually the monitor. The printf function takes one or more arguments, which are enclosed in parentheses and separated by commas. The first argument is a string literal, which is a sequence of characters enclosed in double quotes. The string literal can contain special characters, such as \n for a new line or \t for a tab. The other arguments are optional and can be used to insert values into the string literal using placeholders, such as %d for an integer or %f for a float.
The syntax of the printf() function is:
printf("string literal", optional arguments);
To display “Hello World” on the screen, we can write:
printf("Hello Computer\n");
The \n at the end of the string literal creates a new line after printing the message.
Full code of program
Here is one possible way to do it:
// my frst c program
#include
int main()
{
printf("Hello World\n");
return 0;
}
This program includes the standard input/output header file stdio.h
and defines the main function. The printf
function prints the string “Hello World” followed by a newline character \n
to the standard output. The return 0
statement indicates that the program exits successfully.
The third step is to compile and run our program. To compile our program, we need to use the command line interface of our compiler and specify the name of our source file as an input and the name of our executable file as an output. For example, if we are using GCC compiler on Windows, we can type:
gcc hello.c -o ezyfirst.exe
This command will create an executable file named ezyfirst.exe in the same directory as our source file. After this your output will be displayed.
Hello World
To run our program, we need to execute the executable file from the command line or double-click on it from the file explorer. When we run our program, we should see “Hello Computer” displayed on the screen.
Congratulations! You have successfully written your first C program.