Place Your Query
C Programming
- Control flow-based C Programs
- Enjoy Conditional Programming in C using If...else and switch statement.
- Good, Now write a C program to display "Hello World" on the screen.
- Write a C program to display Your Name, Address and City in different lines.
- C program to find sum of n numbers
- Write a C program For Addition of two numbers using Function.
- Write a Program to Find Simple Interest and Compound Interest.
- Write a Program to Convert Celsius To Fahrenheit and Vice Versa.
- Write a Program to Find Area and Perimeter of Circle, Triangle, Rectangle and Square.
- Write a Program to Swap Two Numbers Using Temporary Variables and Without Using Temporary Variables
- Write a C Program to Design a Simple Menu Driven Calculator
- Simple Expression Based C Programs
- 7. Components of C language
- 1. Introduction to C Programming Language
- 10. Operator Precedence and Associativity
- 11. Comments in C Programming
- 14. Fundamental Control Structure Statements in C [Part-1]
- 15. Fundamental Control Structure Statements in C [Part-2]
- 16. Looping Statements [Fundamental Control Structure Statements in C. #Part-3]
- 17. Keyword break, continue, return and exit [Fundamental Control Structure Statements in C. #Part-4]
- 2. Computer Languages
- 3. Interpreters vs Compilers vs Assemblers in programming languages
- 4. C Program Structure
- 5. Compile and Execute C Program
- 6. Errors in C Program
- 8. C Datatypes
- 9. Operators in C
- Control flow-based C Programs
- Demystifying Bit Masking: Unlocking the Power of Bitwise Operators in C-Language with best Examples.
- 18. Fundamentals of C Functions
- Show Remaining Articles (3)Collapse Articles
Java
AI ML
FAQs
- A Program to find Tokens in C code?
- What are the Common Coding Mistakes.
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
Python Interview Questions
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
17. Keyword break, continue, return and exit [Fundamental Control Structure Statements in C. #Part-4]
All these keywords are used in controlling the program flow. Once they encountered in a program, they work differently as, when a break is observed it skips the further execution of the loop or a switch…case block, whereas when continue is observed it skips the part of the loop and continues the next iteration of the loop with next successive loop control variable-value.
Understanding these keywords and the exit() function is important for controlling the flow of a program, especially within loops and functions. They allow for more precise control over when and how a program should terminate or continue executing.
The break statement:
The break keyword is used to exit a loop prematurely. When the break keyword is encountered within a loop (such as a for, while, or do-while loop), the loop is immediately terminated, and program control is passed to the next statement after the loop.
Syntax:
break;
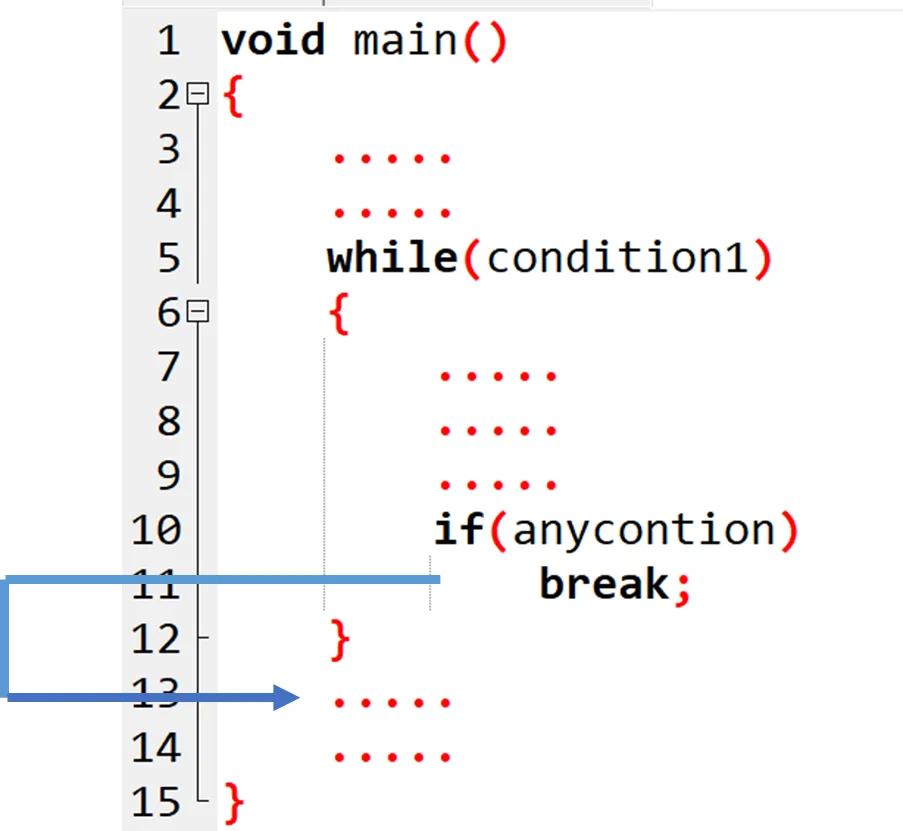
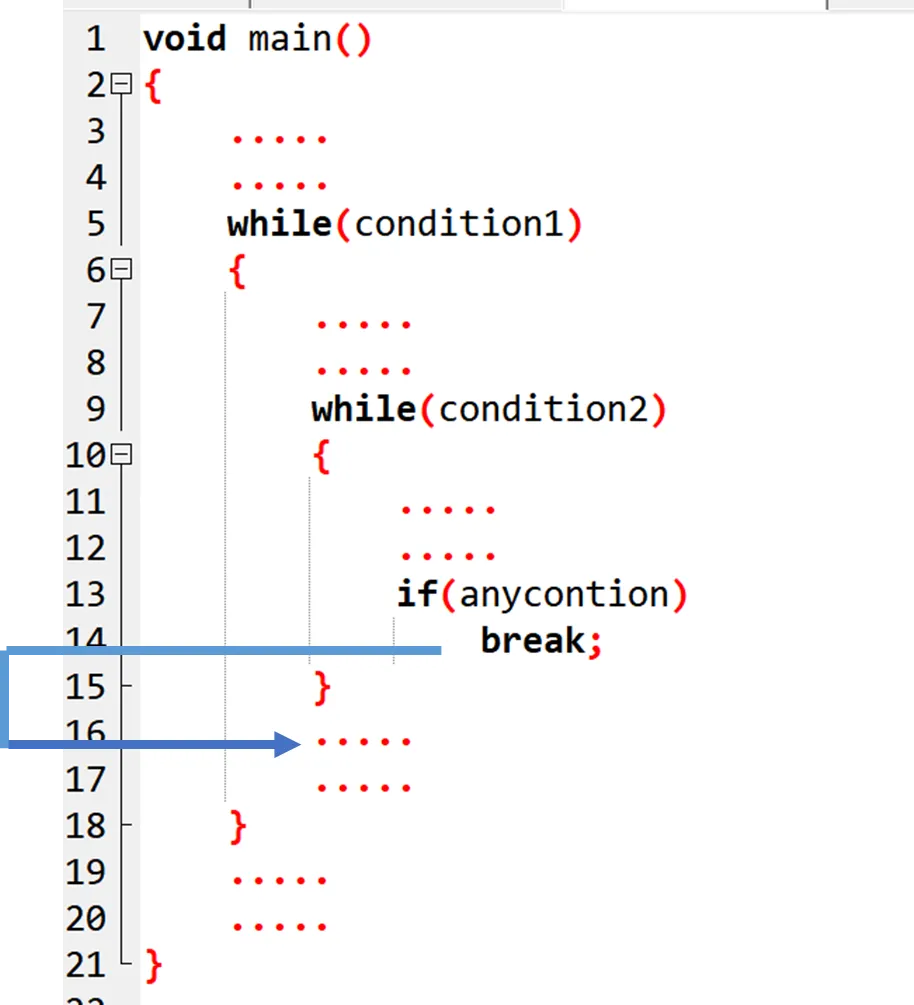
KEY POINTS
- A break statement can appear only inside, or as a body of, a switch statement or a loop.
- The break inside a loop/switch will terminates the loop and the program control is transferred to the next statement after the loop/ /switch.
- The break inside a nested loop, only terminates the execution of the nearest enclosing loop.
- The execution resumes with the statement present next to the terminated loop.
- There is no constraint about the number of break statements that can be present inside a loop.
The continue Statement:
The continue keyword is used to skip the remaining code within a loop and move to the next iteration of the loop. When the continue keyword is encountered within a loop, the current iteration is terminated, and program control goes back to the beginning of the loop to start the next iteration.
Syntax:
continue;
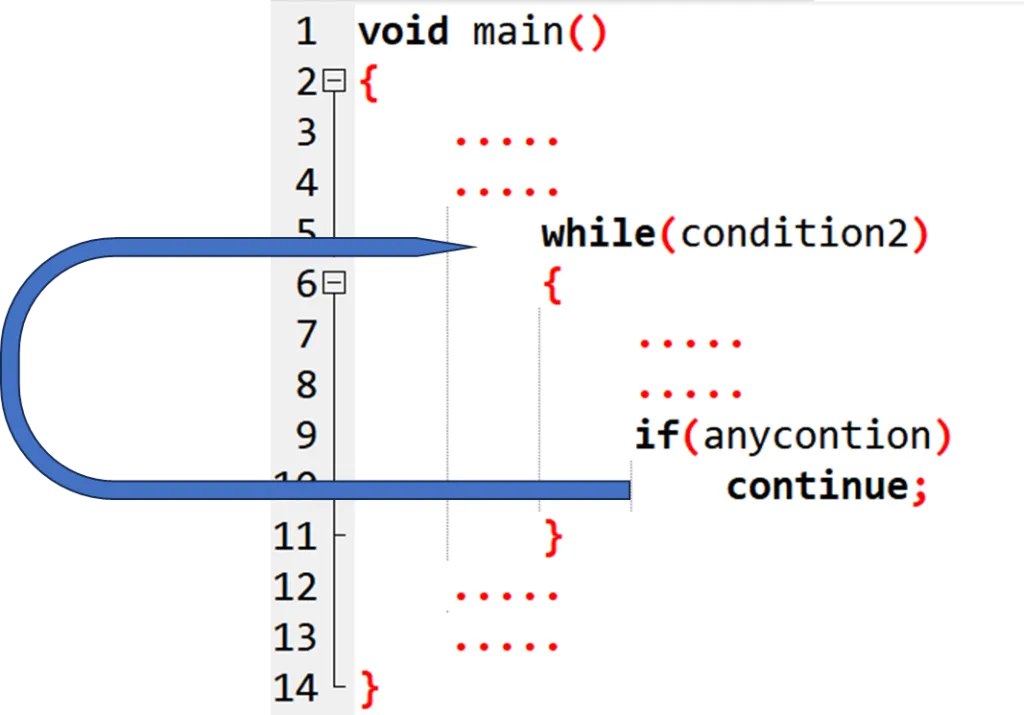
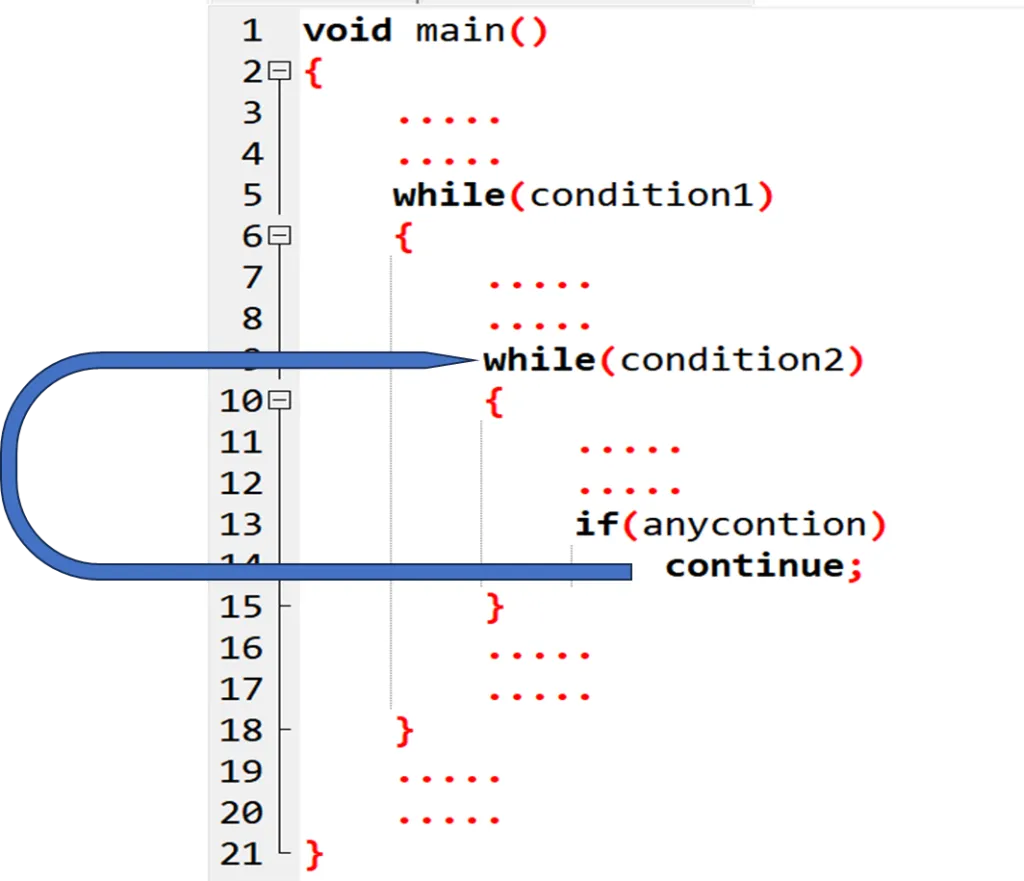
KEY POINTS
- A continue statement can appear only inside, or as the body of, a loop.
- A continue statement terminates the current iteration of the loop.
- When continue statement present inside a nested loop is executed, it only terminates the current iteration of the nearest enclosing loop.
- On the execution of continue statement, the program control is immediately transferred to the header of the loop.
- There is no constraint about the number of continue statements that can be present inside a loop.
Difference between break and continue
Break | Continue |
---|---|
Used to terminate a loop | Used to skip an iteration |
Execution of the loop is terminated immediately | Execution of the current iteration is terminated |
Control is transferred to the statement immediately after the loop | Control is transferred to the beginning of the loop for the next iteration |
Can be used in any loop, switch statement, or nested loop | Can only be used in loops |
Used to exit the loop when a certain condition is met | Used to skip certain iterations when a certain condition is met |
//Example of break :
for (i=0; i<10; i++)
{
if (i == 5)
{ break; }
printf("%d ", i);
}
Output: 0 1 2 3 4
//Example of continue :
for (i=0; i<10; i++)
{
if (i == 5)
{ continue; }
printf("%d ", i);
}
Output: 0 1 2 3 4 6 7 8 9
The return Statement:
The return keyword is used to exit a function and return a value to the calling code. When the return keyword is encountered within a function, the function is immediately terminated, and the specified value is returned to the calling code.
Syntax:
return; <–Form 1
return expression; <–Form 2
return (expression); <–Form 3
KEY POINTS
- A return statement without an expression (i.e. Form 1) can appear only in a function whose return type is void.
- A return statement with an expression (i.e. Form 2 or Form 3) should not appear in a function whose return type is void.
- A return statement terminates the execution of a function and returns the control to the calling function.
The exit() Function
1.include the stdlib.h header file while using the exit () function.
2.It is used to terminate the normal execution of the program while encountered the exit () function.
3.We can use the exit() function to flush or clean all open stream data like read or write with unwritten buffered data.
4.It closses all opened files linked with a parent or another function or file and can remove all files created by the tmpfile function.
5.The program’s behaviour is undefined if the user calls the exit function more than one time.
6.The exit function is categorized into two parts: exit(0) and exit(1).
7.In exit() function the pre defined values for 0 and 1 are EXIT_SUCCESS and EXIT_FAILURE.
Conclusion
- The break keyword: The break keyword is utilized to prematurely exit a loop. Once the break keyword is encountered within a loop (such as a for, while, or do-while loop), the loop will be immediately terminated and program control will be passed to the next statement following the loop.
- The continue keyword: The continue keyword is utilized to bypass the remaining code within a loop and proceed to the next iteration of the loop. When the continue keyword is found within a loop, the current iteration is halted, and program control returns to the beginning of the loop to initiate the next iteration.
- The return keyword: The return statement is used to exit a function and send a value back to the calling code. When the return statement is reached within a function, the function is instantly ended, and the specified value is sent back to the calling code.
- The exit() function: The exit() function is used to terminate the entire program. It is defined in the stdlib.h header file. When the exit() function is called, the program is terminated, and any open files or streams are closed. The function takes an integer argument that represents the exit status of the program, with a value of 0 typically indicating successful termination and non-zero values indicating an error or abnormal termination.