Place Your Query
C Programming
- Control flow-based C Programs
- Enjoy Conditional Programming in C using If...else and switch statement.
- Good, Now write a C program to display "Hello World" on the screen.
- Write a C program to display Your Name, Address and City in different lines.
- C program to find sum of n numbers
- Write a C program For Addition of two numbers using Function.
- Write a Program to Find Simple Interest and Compound Interest.
- Write a Program to Convert Celsius To Fahrenheit and Vice Versa.
- Write a Program to Find Area and Perimeter of Circle, Triangle, Rectangle and Square.
- Write a Program to Swap Two Numbers Using Temporary Variables and Without Using Temporary Variables
- Write a C Program to Design a Simple Menu Driven Calculator
- Simple Expression Based C Programs
- 7. Components of C language
- 1. Introduction to C Programming Language
- 10. Operator Precedence and Associativity
- 11. Comments in C Programming
- 14. Fundamental Control Structure Statements in C [Part-1]
- 15. Fundamental Control Structure Statements in C [Part-2]
- 16. Looping Statements [Fundamental Control Structure Statements in C. #Part-3]
- 17. Keyword break, continue, return and exit [Fundamental Control Structure Statements in C. #Part-4]
- 2. Computer Languages
- 3. Interpreters vs Compilers vs Assemblers in programming languages
- 4. C Program Structure
- 5. Compile and Execute C Program
- 6. Errors in C Program
- 8. C Datatypes
- 9. Operators in C
- Control flow-based C Programs
- Demystifying Bit Masking: Unlocking the Power of Bitwise Operators in C-Language with best Examples.
- 18. Fundamentals of C Functions
- Show Remaining Articles (3)Collapse Articles
Java
AI ML
FAQs
- A Program to find Tokens in C code?
- What are the Common Coding Mistakes.
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
Python Interview Questions
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
8. C Datatypes
In the C programming language, data types refer to a domain of allowed values & the operations that can be performed on those values. The type of a variable determines how much space it occupies in storage and how the bit pattern stored is interpreted. There are 4 fundamental (primitive) C datatypes, which are- char, int, float &, double. Char is used to store any single character; int is used to store any integer value, float is used to store any single precision floating point number & double is used to store any double precision floating point number.
You can use two qualifiers to expand the range of these basic types.
- a. Sign qualifier– signed & unsigned
- b. Size qualifier- short & long
Why to Use of Data Types?
- To identify the type of a variable when it declared.
- To identify the type of the return value of a function.
- To identify the type of a parameter expected by a function.
ANSI C provides three types of data types:
- Primary(Built-in) Data Types:
void, int, char, double and float. - Derived Data Types:
Array, References, and Pointers. - User Defined Data Types:
Structure, Union, and Enumeration.
Classification Chart
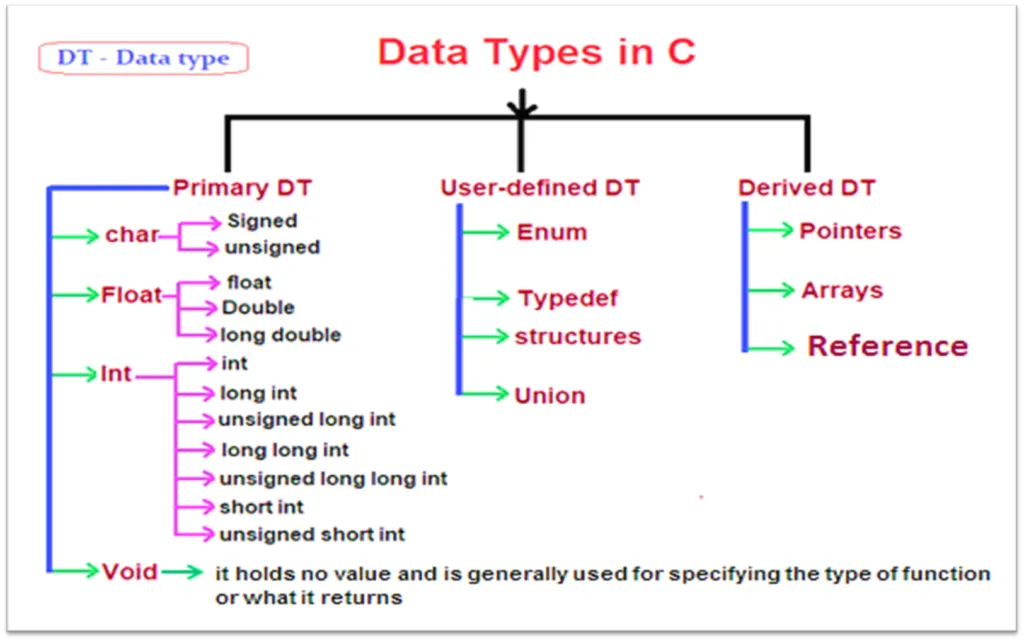
Primitive Datatypes
Type | Storage size | Format | Value range |
char | 1 byte | %c | -128 to 127 |
unsigned char | 1 byte | %c | 0 to 255 |
int | 4 bytes | %d | -2,147,483,648 to 2,147,483,647 |
unsigned int | 4 bytes | %u | 0 to 4,294,967,295 |
short | 2 bytes | %d | -32,768 to 32,767 |
unsigned short | 2 bytes | %u | 0 to 65,535 |
long | 4 bytes | %ld | -2,147,483,648 to 2,147,483,647 |
unsigned long | 4 bytes | %lu | 0 to 4,294,967,295 |
Type | Storage size | Format | Value range | Precision |
---|---|---|---|---|
float | 4 byte | %f | 1.2E-38 to 3.4E+38 | 6 decimal places |
double | 8 byte | %lf | 2.3E-308 to 1.7E+308 | 15 decimal places |
long double | 10 byte | %LF | 3.4E-4932 to 1.1E+4932 | 19 decimal places |
void datatype | As the name suggests it holds no value and is generally used for specifying the type of function or what it returns. If the function has a void type, it means that the function will not return any value. |
Type | Description |
---|---|
Function returns as void | A function with no return value has the return type as void. For example, void exit (int status); |
Function arguments as void | A function with no parameter can accept a void. For example, int rand(void); |
Pointers to void | A pointer of type void * represents the address of an object, but not its type. For example, a memory allocation function void *malloc( size_t size ); This returns a pointer to void which can be casted to any data type. |
Three more data types have been added in C99:
- _Bool
- _Complex
- _Imaginary
Declaration of Primary Data Types with Variable Names
After taking suitable variable names, they need to be assigned with a data type. This is how the data types are used along with variables:
Examples of Primitive datatypes
Example 1:
int age;
char letter;
float height, width;
char answer, choice;
Example 2:
#include
int main()
{
int a = 4000; // positive integer data type
float b = 5.2324; // float data type
char c = 'Z'; // char data type
long d = 41657; // long positive integer data type
long e = -21556; // long -ve integer data type
int f = -185; // -ve integer data type
short g = 130; // short +ve integer data type
short h = -130; // short -ve integer data type
double i = 4.1234567890; // double float data type
float j = -3.55; // float data type
}
Difference between Float and Double Data Types
float Datatype | double Datatype |
---|---|
Float takes 4 bytes for storage. | Double takes 8 bytes for storage. |
A value having a range within 1.2E-38 to 3.4E+38 can be assigned to float variables. | A value having range within 2.3E-308 to 1.7E+308 can be assigned to double type variables |
Has a precision of 6 decimal places. | Has a precision of 15 decimal places. |
It has single precision. | It has the double precision or you can say two times more precision than float. |
This is generally used for graphic based libraries for making the processing power of your programs faster, as it is simpler to manage by compilers. | This is the most commonly used data type in programming languages for assigning values having a real or decimal based number within, such as 3.14 for pi. |
Derived Data Types
C language supports three derived datatypes
Data Types | Description |
---|---|
Arrays | Arrays are sequences of data items having homogeneous values. They have adjacent memory locations to store values. |
Pointers | These are used to access the memory and deal with their addresses. |
References | Function pointers allow referencing functions with a particular signature. |
User Defined Data Types
C datatypes allows the feature called type definition which allows programmers to define their identifier that would represent an existing data type.
Data Types | Description |
Structure | It is a package of variables of different types under a single name. “struct” keyword is used to define a structure. |
Union | These allow storing various data types in the same memory location. Programmers can define a union with different members, but only a single member can contain a value at given time. |
Enum | Enumeration is a special data type that consists of integral constants, and each of them is assigned with a specific name. “enum” keyword is used to define the enumerated data type. |
Write a program to check a datatype size.
The storage representation and machine instructions vary from one machine to another. The sizeof operator can be used to obtain the precise size of a type or variable on a specific platform. On some platforms, the size of a type or variable may be different due to factors such as the underlying architecture, compiler, and operating system. It is important to consider these differences when writing code that needs to be portable across different machines.
// Program to check the datatype or its variable size
// use keyword sizeof to find a size.
#include
#include
int main() {
printf("Storage size for void : %d \n", sizeof(void));
printf("Storage size for char : %d \n", sizeof(char));
printf("Storage size for float : %d \n", sizeof(float));
printf("Storage size for double : %d \n", sizeof(double));
printf("Storage size for int : %d \n", sizeof(int));
printf("Storage size for long int : %d \n", sizeof(long int));
printf("Storage size for unsigned int : %d \n", sizeof(unsigned int));
printf("Storage size for unsigned long int : %d \n", sizeof(unsigned long int));
printf("Storage size for long double : %d \n", sizeof(long double));
printf("Minimum long double negative value: %E\n", LDBL_MIN );
printf("Maximum long double positive value: %E\n", LDBL_MAX );
printf("Precision value: %d\n", LDBL_DIG );
return 0;
}
Output
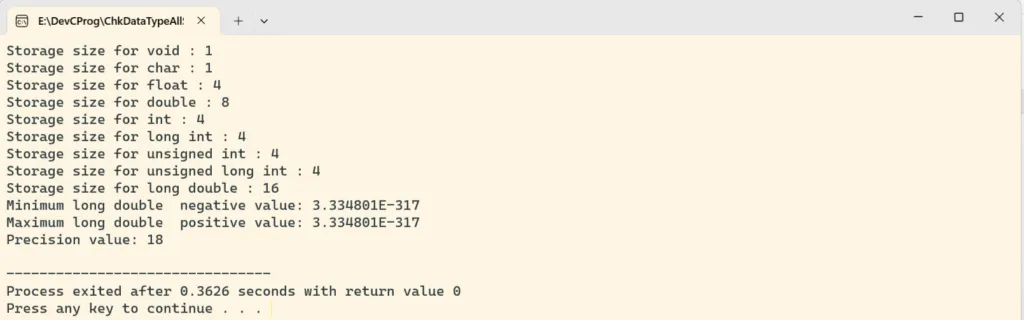