Place Your Query
C Programming
- Control flow-based C Programs
- Enjoy Conditional Programming in C using If...else and switch statement.
- Good, Now write a C program to display "Hello World" on the screen.
- Write a C program to display Your Name, Address and City in different lines.
- C program to find sum of n numbers
- Write a C program For Addition of two numbers using Function.
- Write a Program to Find Simple Interest and Compound Interest.
- Write a Program to Convert Celsius To Fahrenheit and Vice Versa.
- Write a Program to Find Area and Perimeter of Circle, Triangle, Rectangle and Square.
- Write a Program to Swap Two Numbers Using Temporary Variables and Without Using Temporary Variables
- Write a C Program to Design a Simple Menu Driven Calculator
- Simple Expression Based C Programs
- 7. Components of C language
- 1. Introduction to C Programming Language
- 10. Operator Precedence and Associativity
- 11. Comments in C Programming
- 14. Fundamental Control Structure Statements in C [Part-1]
- 15. Fundamental Control Structure Statements in C [Part-2]
- 16. Looping Statements [Fundamental Control Structure Statements in C. #Part-3]
- 17. Keyword break, continue, return and exit [Fundamental Control Structure Statements in C. #Part-4]
- 2. Computer Languages
- 3. Interpreters vs Compilers vs Assemblers in programming languages
- 4. C Program Structure
- 5. Compile and Execute C Program
- 6. Errors in C Program
- 8. C Datatypes
- 9. Operators in C
- Control flow-based C Programs
- Demystifying Bit Masking: Unlocking the Power of Bitwise Operators in C-Language with best Examples.
- 18. Fundamentals of C Functions
- Show Remaining Articles (3)Collapse Articles
Java
AI ML
FAQs
- A Program to find Tokens in C code?
- What are the Common Coding Mistakes.
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
Python Interview Questions
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
9. Operators in C
Assignment Operators
The assignment operator is responsible for assigning values to the variables. While the equal sign (=) is the fundamental assignment operator, C also supports other assignment operators that provide shorthand ways to represent common variable assignments. They are shown in the table. Assignment operators are used to assign the result of an expression to a variable. C has a variety of shorthand assignment operators.
OPERATOR | SYNTAX | EQUIVALENT TO |
/= | variable /= expression | variable = variable / expression |
\= | variable \= expression | variable = variable \ expression |
*= | variable *= expression | variable = variable * expression |
+= | variable += expression | variable = variable + expression |
-= | variable -= expression | variable = variable – expression |
&= | variable &= expression | variable = variable & expression |
^= | variable ^= expression | variable = variable ^ expression |
<<= | variable <<= amount | variable = variable << amount |
>>= | variable >>= amount | variable = variable >> amount |
Logical Operators
Logical operators are used to evaluate two or more conditions. In General, Logical operators are used to combine relational expressions, but they are not limited to just relational expression you can use any kind of expression even constants. If the result of the logical operator is true then1
is returned otherwise0
is returned. C language supports three logical operators. They are- Logical AND (&&), Logical OR (||) and Logical NOT (!).
As in case of arithmetic expressions, the logical expressions are evaluated from left to right.
Operator | Description | Example |
---|---|---|
&& | Called Logical AND operator. If both the operands are non-zero, then the condition becomes true. | (A && B) is false. |
|| | Called Logical OR Operator. If any of the two operands is non-zero, then the condition becomes true. | (A || B) is true. |
! | Called Logical NOT Operator. It is used to reverse the logical state of its operand. If a condition is true, then Logical NOT operator will make it false. | !(A && B) is true. |
A | B | A &&B |
0 | 0 | 0 |
0 | 1 | 0 |
1 | 0 | 0 |
1 | 1 | 1 |
A | B | A || B |
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 1 |
A | ! A |
0 | 1 |
1 | 0 |
Example: Program to check logical operators
//Sample program to check logical operators
#include
int main() {
int a = 10;
int b = 20;
int c ;
if ( a && b ) {
printf("Statement 1 - Status of Condition is true\n" );
}
if ( a || b ) {
printf("Statement 2 - Status of Condition is true\n" );
}
/* Now change the value of a and b */
a = 0;
b = 10;
if ( a && b ) {
printf("Statement 3 - Status of Condition is true\n" );
} else {
printf("Statement 3 - Status of Condition is not true\n" );
}
if ( !(a && b) ) {
printf("Statement 4 - Status of Condition is true\n" );
}
return 0;
}
Output
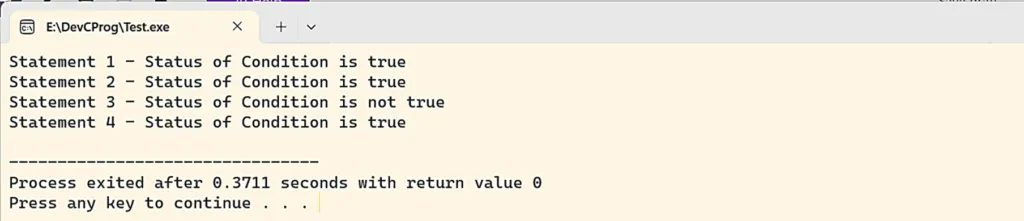
Example 1:
int age = 10, height = 45;
(age < 12 && height < 48) || (age > 65 && height > 72);
Solution: In this case, the order of evaluation of operators will be parentheses (()
), relational operators (<
,>
), AND (&&
) operator, OR (||
) operator.
(age < 12 && height < 48) || (age > 65 && height > 72)
=> (10 < 12 && 45 < 48) || (10 > 65 && 45 > 72)
=> (1 && 1) || (10 > 65 && 45 > 72) => 1 || (10 > 65 && 45 > 72)
=> 1 || (0 && 0)
=> 1 || 0
=> 1
Example 2:
int year = 2000;
(year % 4 == 0 && year % 100 != 0 ) || (year % 400 == 0);
Solution: In this case, the order of evaluation of operators will be parentheses (()
), Modular division (%
), Relational operators (==
,!=
) AND (&&
) operator, OR (||
) operator.
(year % 4 == 0 && year % 100 != 0 ) || (year % 400 == 0)
=> (2000 % 4 == 0 && 2000 % 100 != 0 ) || (2000 % 400 == 0)
=> (0 == 0 && 2000 % 100 != 0 ) || (2000 % 400 == 0)
=> (0 == 0 && 0 != 0 ) || (2000 % 400 == 0)
=> (1 && 0 != 0 ) || (2000 % 400 == 0)
=> (1 && 0 ) || (2000 % 400 == 0)
=> 0 || (2000 % 400 == 0)
=> 0 || (0 == 0)
=> 0 || 1
=> 1