Place Your Query
C Programming
- Control flow-based C Programs
- Enjoy Conditional Programming in C using If...else and switch statement.
- Good, Now write a C program to display "Hello World" on the screen.
- Write a C program to display Your Name, Address and City in different lines.
- C program to find sum of n numbers
- Write a C program For Addition of two numbers using Function.
- Write a Program to Find Simple Interest and Compound Interest.
- Write a Program to Convert Celsius To Fahrenheit and Vice Versa.
- Write a Program to Find Area and Perimeter of Circle, Triangle, Rectangle and Square.
- Write a Program to Swap Two Numbers Using Temporary Variables and Without Using Temporary Variables
- Write a C Program to Design a Simple Menu Driven Calculator
- Simple Expression Based C Programs
- 7. Components of C language
- 1. Introduction to C Programming Language
- 10. Operator Precedence and Associativity
- 11. Comments in C Programming
- 14. Fundamental Control Structure Statements in C [Part-1]
- 15. Fundamental Control Structure Statements in C [Part-2]
- 16. Looping Statements [Fundamental Control Structure Statements in C. #Part-3]
- 17. Keyword break, continue, return and exit [Fundamental Control Structure Statements in C. #Part-4]
- 2. Computer Languages
- 3. Interpreters vs Compilers vs Assemblers in programming languages
- 4. C Program Structure
- 5. Compile and Execute C Program
- 6. Errors in C Program
- 8. C Datatypes
- 9. Operators in C
- Control flow-based C Programs
- Demystifying Bit Masking: Unlocking the Power of Bitwise Operators in C-Language with best Examples.
- 18. Fundamentals of C Functions
- Show Remaining Articles (3)Collapse Articles
Java
AI ML
FAQs
- A Program to find Tokens in C code?
- What are the Common Coding Mistakes.
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
Python Interview Questions
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
9. Operators in C
Bitwise Operators
- Bitwise operators perform operations at bit level. These operators include: bitwise AND, bitwise OR, bitwise XOR and shift operators.
- The bitwise AND operator (&) is a small version of the boolean AND (&&) as it performs operation on bits instead of bytes, chars, integers, etc.
- The bitwise OR operator (|) is a small version of the boolean OR (||) as it performs operation on bits instead of bytes, chars, integers, etc.
- The bitwise NOT (~), or complement, is a unary operation that performs logical negation on each bit of the operand. By performing negation of each bit, it actually produces the ones’ complement of the given binary value.
- The bitwise XOR operator (^) performs operation on individual bits of the operands.
- The result of all bitwise AND, OR and XOR operations are shown in the table below.
Operator | Description |
& (Binary AND Operator) | If a bit exists in the two operands, it copies a bit to the result. |
| (Binary OR Operator.) | Only if the bit exists in one of the operands, it copies a bit. |
^ (Binary XOR Operator) | If a bit is set in one operand rather than both, it copies the bit. |
~ (Binary One’s Complement Operator) | It is a unary operator that has the effect of ‘flipping’ bits. |
<< (Binary Left Shift Operator) | It moves the value of the right operand to the left by the number of bits specified by the right operand. |
>> (Binary Right Shift Operator) | It moves the value of the left operands to the right by the number of bits mentioned by the right operand. |
Bitwise Operator | Description | Example Let A= 60, i.e 0011 1100 |
---|---|---|
~ | Binary Ones Complement Operator is unary and has the effect of ‘flipping’ bits. | (~A ) = -60, i.e,. 11000011 in 1’s complement form. ————————————– To get -60, add 1 to above i.e (~A ) = -60, i.e,. 1100 0100 in 2’s complement form. |
<< | Binary Left Shift Operator. The left operands value is moved left by the number of bits specified by the right operand. | A << 2 = 240 i.e., 1111 0000 |
>> | Binary Right Shift Operator. The left operands value is moved right by the number of bits specified by the right operand. | A >> 2 = 15 i.e., 0000 1111 |
p | q | p & q (Bitwise AND) | p | q (Bitwise OR) | p ^ q (Bitwise XOR) |
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
1 | 0 | 0 | 1 | 1 |
Special operators
Besides the operators mentioned above in C, some more operators are used to carry out different tasks. Let’s have a look at them:
Operator | Description | Example |
---|---|---|
sizeof() | Returns the size of a variable. | sizeof(a), where a is integer, will return 4. |
& | Returns the address of a variable. | &a; returns the actual address of the variable. |
* | Pointer to a variable. | *a; |
? : | Conditional Expression. | If Condition is true ? then value X : otherwise value Y |
sizeof Operator
The sizeof is a unary operator in the C language used to calculate the size of operands. Its result is an unsigned internal type represented by size_t.
#include
int main()
{ int i=10,a;
printf("integer: %d\n", sizeof(a));
return 0;
}
// Output :
// Integer 4
Comma Operator
The comma operator in C is represented by the token. It is a binary operator with the lowest precedence among all C operators. It works as an operator and separator. It evaluates the first operand, discards the result, evaluates the second operand, and returns its value.
int a = 1, b = 2, c = 3;
int result = (a++, b++, c++);
/* The value of result will be 3,
as the comma operator evaluates a, b, and c
in sequence and returns the value of c. */
Conditional Operator
It is written in the form of Expression1? Expression2: Expression3. Expression 1 is the condition that needs to be evaluated, and if it is true, then Expression 2 is executed, and we return the result.
However, if Expression 1 is false, we execute and return the result of Expression 3. We can use conditional operators to replace if…else statements.
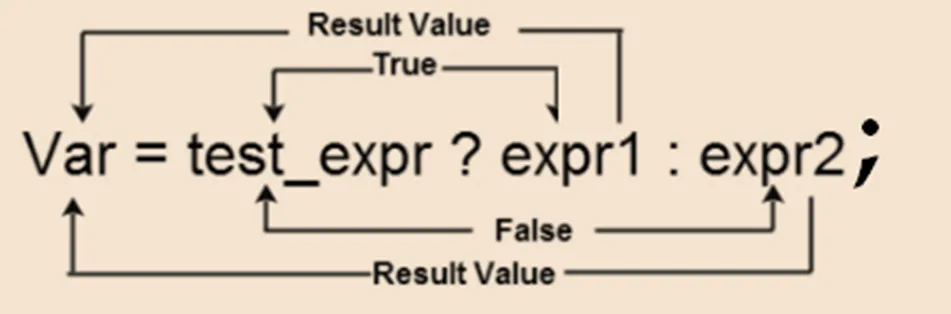
// Program to find maximum in first two and maximum in all three input values to a,b,c
#include
void main() {
int x, y, z, Max1,Max2,Min;
printf("Enter three whole numbers:\n");
scanf( "%d%d%d",&x, &y, &z ) ;
Min = x < y ? x : y;
printf("Minimum of the first two numbers= %d\n" ,Min );
Max1 = (x > y? x : y);
printf("Maximum of the first two numbers= %d\n" ,Max1);
Max2 = (x> y ?x : y) > z ? (x>y ? x:y): z;
printf("Maximum of the three numbers is= %d\n", Max2);
}
dot (.) and arrow (->) Operators
They are member operators used to reference individual members of classes, unions, and structures. The dot (.) operator is applied to real objects, while the arrow operator (->) is used with a pointer.
Cast Operator
It converts one type of data to another type. This special C operator forces one data type to convert into another. The process of conversion may result in loss of data precision or information, so it is important to use type casting carefully and consider the implications of the conversion. As an example, if you are converting a floating-point number to an integer, you may lose the decimal part of the number.
&,* Operator
& returns a variable’s address while * operator is a pointer to a variable. For example, &a; will give the address of the variable, and *var will be a pointer to a variable ‘var’. This will be discussed in detail in the topic of pointers. In C programming, pointers are widely used to work with memory addresses and manipulate data at a lower level. Understanding pointers is crucial for writing efficient and optimized code.