Place Your Query
C Programming
- Control flow-based C Programs
- Enjoy Conditional Programming in C using If...else and switch statement.
- Good, Now write a C program to display "Hello World" on the screen.
- Write a C program to display Your Name, Address and City in different lines.
- C program to find sum of n numbers
- Write a C program For Addition of two numbers using Function.
- Write a Program to Find Simple Interest and Compound Interest.
- Write a Program to Convert Celsius To Fahrenheit and Vice Versa.
- Write a Program to Find Area and Perimeter of Circle, Triangle, Rectangle and Square.
- Write a Program to Swap Two Numbers Using Temporary Variables and Without Using Temporary Variables
- Write a C Program to Design a Simple Menu Driven Calculator
- Simple Expression Based C Programs
- 7. Components of C language
- 1. Introduction to C Programming Language
- 10. Operator Precedence and Associativity
- 11. Comments in C Programming
- 14. Fundamental Control Structure Statements in C [Part-1]
- 15. Fundamental Control Structure Statements in C [Part-2]
- 16. Looping Statements [Fundamental Control Structure Statements in C. #Part-3]
- 17. Keyword break, continue, return and exit [Fundamental Control Structure Statements in C. #Part-4]
- 2. Computer Languages
- 3. Interpreters vs Compilers vs Assemblers in programming languages
- 4. C Program Structure
- 5. Compile and Execute C Program
- 6. Errors in C Program
- 8. C Datatypes
- 9. Operators in C
- Control flow-based C Programs
- Demystifying Bit Masking: Unlocking the Power of Bitwise Operators in C-Language with best Examples.
- 18. Fundamentals of C Functions
- Show Remaining Articles (3)Collapse Articles
Java
AI ML
FAQs
- A Program to find Tokens in C code?
- What are the Common Coding Mistakes.
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
Python Interview Questions
- Easy Learning, Python QAs for Beginner’s to Pro Part-1
- Easy Learning, Python QAs for Beginner’s to Pro Part-2
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-1
- Easy Learning, Python Strings QAs for Beginner’s to Pro Part-2
- Easy Learning, Python String Functions QAs for Beginner to Pro Part-3
- Python Dictionary
9. Operators in C
C operators are symbols that are used to perform mathematical or logical manipulations. C programming language is rich with built-in operators.
C is a widely used programming language with many built-in operators for carrying out various actions and tasks as per program needs. An operator in C plays a role in manipulating data and performing operations on variables and values.
These symbols are operators, each representing a particular operation to be carried out on operands. They are used in conditional, mathematical, and logical expressions.
There is a wide range of operators in the C language; each belongs to a different category according to its functionalities.
Operators in C language take part in a program for manipulating data and variables and it also form a part of the mathematical or logical expressions.
Quick Access
Types of Operators in C
- Arithmetic Operators
- Increment and Decrement Operators
- Relational Operators
- Logical Operators
- Assignment Operators
- Conditional Operator
- Bitwise Operators
- Special Operators
Arithmetic Operators
Arithmetic operations are used for mathematical or arithmetic calculations, such as division (/), addition (+), subtraction (-), multiplication (*), etc., on operands. It performs operations on constants and variables, i.e., numerical values.
OPERATION | OPERATOR | SYNTAX | COMMENT | RESULT |
Multiply | * | a * b | result = a * b | 27 |
Divide | / | a / b | result = a / b | 3 |
Addition | + | a + b | result = a + b | 12 |
Subtraction | – | a – b | result = a – b | 6 |
Modulus | % | a % b | result = a % b | 0 |
//Example:
#include
int main()
{ int i=3,j=7,k;
k=i+j;
printf("sum of two numbers is %d\n", k);
return 0;
}
Output

Increment and Decrement Operators
- Increment and Decrement Operators are useful operators generally used to minimize the calculation, for example.
- ++x & x++ this means x=x+1
- or
- –x & x−− this means x=x-1.
- But there is difference between ++ or −− written before or after the operand.
- Applying the pre-increment first add one to the operand and then the result is assigned to the variable on left.
- whereas post-increment first assigns the value to the variable on the left and then increment the operand.
Operator | Description |
++ | Increment |
−− | Decrement |
Increment and decrement operators are exclusively used with variables and cannot be applied to constants or expressions.
//let us say:
int x = 5;
x++; // Now x is 6
x--;// Now x is 5
// a valid and invald use of pre/post inc/decrement operator
int x = 1, y = 1;
++x; // valid
++5; // invalid - increment operator operating on a constant value
++(x+y); // invalid - increment operating on an expression
Example
#include
int main()
{
int x=5, y=7;
printf(“ the pre increment operation %d”, ++x); // first increment , then print
printf(“ the pre increment operation %d”, ++y); // first increment , then print
printf(“ the post increment operation %d”, x++); // first print, then increment
printf(“ the post increment operation %d”, y++); // first print, then increment
printf(“ the post increment operation %d”, x++); // first print, then increment
return 0;
}
Output
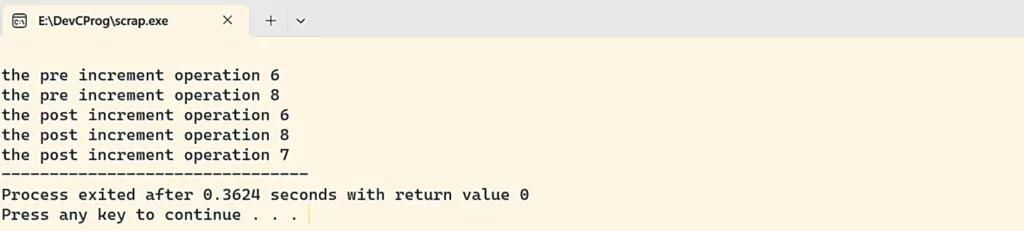
Relational Operators
Relational operators in C compare the values of two operands in a program to determine their relationship. If the relationship is true, it returns 1; otherwise, it returns 0. These operators are commonly used for decision-making and loop operations.
For example, checking if one operand is greater or equal to another. Relational operators include ==, >= , <= .
OPERATOR | MEANING | EXAMPLE |
< | LESS THAN | 3 < 5 GIVES 1 |
> | GREATER THAN | 7 > 9 GIVES 0 |
>= | LESS THAN OR EQUAL TO | 100 >= 100 GIVES 1 |
<= | GREATER THAN EQUAL TO | 50 >=100 GIVES 0 |
== | RETURNS 1 IF BOTH OPERANDS ARE EQUAL, 0 OTHERWISE | (4 == 9) is not true. |
!= | RETURNS 1 IF OPERANDS DO NOT HAVE THE SAME VALUE, 0 OTHERWISE | (4 != 9) is true. |
Example: Program to check role of relational operators
// sample code to check role of relational operators
// By milndbhatt
#include
int main()
{
int a = 10, b = 20;
printf("a = %d\n", a);
printf("b = %d\n\n", b);
// Check a is greater than b.
printf("a > b : %d\n", a > b);
// Check a is greater than or equal to b.
printf("a >= b : %d\n", a >= b);
// Check a is smaller than b.
printf("a < b : %d\n", a < b);
// Check a is smaller than or equal to b.
printf("a <= b : %d\n", a <= b);
// Check a is equal to b.
printf("a == b : %d\n", a == b);
// Check a is not equal to b.
printf("a != b : %d\n", a != b);
return 0;
}
Output
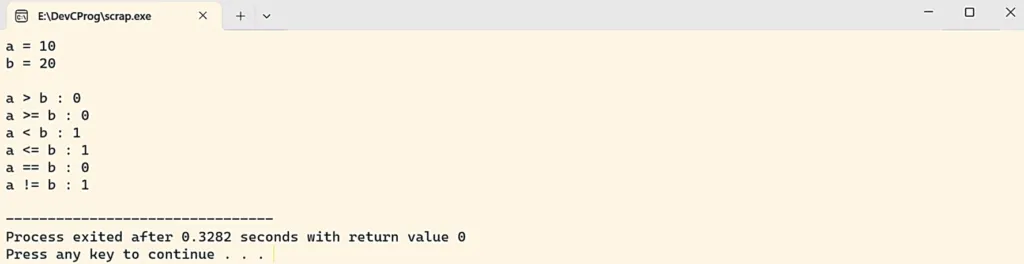